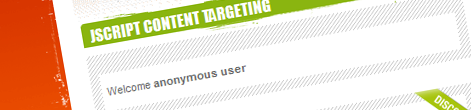
No-code content targeting in SharePoint 2010
No-code SharePoint solutions became very popular in the last two years. Having virtually no deployment boundaries they are ideal for power users to enhance their sites with new dynamic functionality. While SharePoint 2007 already allowed us to do some great stuff with no-code solutions, thanks to the JavaScript Object Model provided with SharePoint 2010 we can make even richer solution without a single line of server-side code. And while retrieving information from SharePoint is pretty cool, being able to target it to your users is what it takes to use no-code solutions on Internet-facing websites.
Target your content
Targeting content based on the authentication state of the user(anonymous/logged in) used to be something for server-side code only. Using either a custom control or the LoginView ASP.NET control you could wrap pieces of page and conditionally display it to the user depending whether he was logged in or not. This was a great solution to include some extra CSS or JavaScript files for anonymous users or do some other trickery frequently used on Internet-facing websites.
Recently no-code SharePoint solutions gained popularity. The great thing about them is that they can be quickly deployed by power users without any help of the IT department. Especially using the new JavaScript Object Model provided with SharePoint 2010 you can create really powerful solutions without a single line of server-side code. So with all that SharePoint data available in JavaScript it can be very useful to be able to display it conditionally if the user has logged in or is still anonymous. Let me show you how.
Important: frequently conditional content based on the authentication state is being used to secure some information from anonymous users. While there’s nothing wrong to do that while using server-side code, you should never work with sensitive data while targeting content with JavaScript. Because JavaScript is being executed in the browser the sensitive information could be easily intercepted introducing a security risk.
Targeting content in SharePoint 2010 with JavaScript
The following code snippet contains a generic JavaScript function that allows you to conditionally display content to anonymous and authenticated users.
Type.registerNamespace("Mavention.Audiences");
Mavention.Audiences.showTargetedContent = function (anonymousCallback, authenticatedCallback) {
Mavention.Audiences.anonymousCallback = anonymousCallback;
Mavention.Audiences.authenticatedCallback = authenticatedCallback;
if (!Mavention.Audiences.user) {
var context = SP.ClientContext.get_current();
Mavention.Audiences.user = context.get_web().get_currentUser();
context.load(Mavention.Audiences.user);
context.executeQueryAsync(function () {
var callback = Mavention.Audiences.user.get_loginName() != null ? Mavention.Audiences.authenticatedCallback : Mavention.Audiences.anonymousCallback;
if (callback != null) {
callback.call();
}
},
function () {
if (Mavention.Audiences.anonymousCallback != null) {
Mavention.Audiences.anonymousCallback.call();
}
});
}
else {
var callback = Mavention.Audiences.user.get_loginName() != null ? Mavention.Audiences.authenticatedCallback : Mavention.Audiences.anonymousCallback;
if (callback != null) {
callback.call();
}
}
}
The showTargetedContent function takes two parameters which are callback methods that will be called after checking if the current user is authenticated or not. Those methods can do anything from displaying a message box with a welcome message to triggering some more sophisticated flow within your solution. As an example we will display a welcome message containing the name of the current user or anonymous user if the user hasn’t logged in yet.
Using the showTargetedContent function is very simple:
Mavention.Audiences.showTargetedWelcomeMessage = function () {
Mavention.Audiences.showTargetedContent(function () {
Mavention.Audiences.showWelcomeMessage();
}, function () {
Mavention.Audiences.showWelcomeMessage(Mavention.Audiences.user.get_title());
});
}
Mavention.Audiences.showWelcomeMessage = function (userName) {
if (!userName) {
userName = 'anonymous user';
}
document.getElementById('mv-WelcomeMessage').innerHTML = '<p>Welcome <strong>' + userName + '</strong></p>';
}
SP.SOD.executeOrDelayUntilScriptLoaded(Mavention.Audiences.showTargetedWelcomeMessage, 'sp.js');
First we wrap the call to the showTargetedContent function in a simple function (showTargetedWelcomeMessage in this example). This has to be done since the showTargetedContent function depends on the SharePoint JSOM which is being loaded on demand and doesn’t necessarily has to be available on the first call of the method. To ensure that the code will run correctly we can use the SP.SOD.executeOrDelayUntilScriptLoaded function which allows us to wait with the execution of a function until the required script has loaded. The showWelcomeMessage function is a simple helper function that displays a welcome message in a div somewhere on the page.
After adding the above JavaScript snippet to a page: either directly in Page Layout or in a Content Editor Web Part you can see the welcome message that is different for anonymous and authenticated users:
How it works
The first thing that the showTargetedContent function does is to check if the details about the current user are already available. While they’re empty on the first call, they are available on all subsequent calls. Since the user information cannot change without reloading the page it’s a good practice to reuse them if you’re having multiple calls to this function on the page.
The first time time showTargetedContent function is being called it requests user information from SharePoint using the JSOM API. The information about the user is being stored in the Mavention.Audiences.user property for future reuse. While requesting the data two callback methods are passed. The first is being triggered if the query succeeds and the other one if it fails. Using the information about the current user we can easily determine if the current user has logged in or if he’s still anonymous.
Depending on the authentication state of the user one of the callback methods is being used and it will be executed if it’s not empty.
Summary
SharePoint 2010 ships with rich JavaScript Object Model that allows you to create rich no-code solutions. Targeting content based on the authentication state of the user gives you the ability to enhance the functionality of your Internet-facing website built on the SharePoint 2010 platform without the complexity of a full deployment of a custom SharePoint Farm Solution even further.
Technorati Tags: SharePoint 2010,WCM,JavaScript