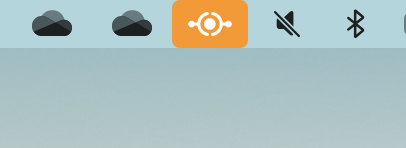
Add background color to menu bar icon in macOS
When building apps for macOS, you might want to add a background color to your icon in the menu bar. This is a great way to make your app stand out and make it easier for users to find your app in the menu bar.
To add a background color to your icon in the menu bar, the trick is to find the right view to apply the background color to. Here’s how you can do it in Swift.
Start, by getting a reference to the status item. Then, get the status item’s button. Configure the image to fit the button.
// get a reference to the status item
let statusItem = NSStatusBar.system.statusItem(withLength: NSStatusItem.squareLength)
// get the status item's button
let button = statusItem.button
// configure the image to fit the button
button?.imageScaling = NSImageScaling.scaleProportionallyUpOrDown
Next, get the view behind the button and apply the background color to it. Set the image on the button. To align the button with standard macOS buttons such as microphone, camera or screen recording, configure the corners to be rounded.
// this is the view you need to get
let buttonView = button?.superview?.window?.contentView
// set the image on the button
button.image = NSImage(named:NSImage.Name("ProxyEnabledWhite"))
// set the background color
buttonView.layer?.backgroundColor = NSColor.systemOrange.cgColor
// round the corners
buttonView.layer?.cornerRadius = 4
If you get the wrong view, the background color will overlay the image. Also, configure the template-rendering-intent
of the image to show on the button as original
. This will ensure that the image is not affected by the background color and has enough contrast.
Here’s the result:
To clear the background color, change the backgroundColor
to NSColor.clear
. Change the image configure as a template image so that it automatically adjusts to the system appearance. If you need, you can also choose to display the image as disabled.
buttonView.layer?.backgroundColor = NSColor.clear.cgColor
button.image = NSImage(named:NSImage.Name("ProxyEnabledBlack"))
button.appearsDisabled = true
Here’s the result:
Summary
Adding a background color to your icon in the menu bar on macOS is a great way to make your app stand out and make it easier for users to find your app in the menu bar. By applying the background color to the right view, you can combine it with your icon and align it with standard macOS buttons such as microphone, camera or screen recording.