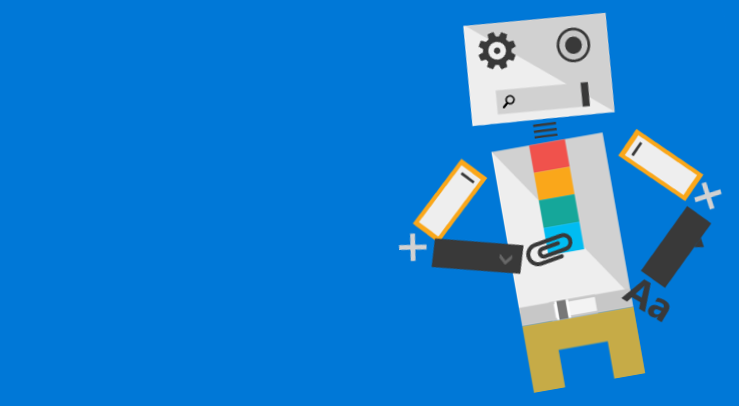
Build Angular applications with style - the table component (ngOfficeUIFabric)
If you’re building Angular applications on the Office platform, you might have heard about the Angular directives for Office UI Fabric. Did you know however that the directives offer more than just great UI?
Developing on the Office platform in style
Over the last few years Microsoft heavily invested in the developer opportunities on the Office platform. We’ve come a long way from proprietary development models to leveraging the most commonly used web frameworks.
As the development on the Office platform simplified and more and more solutions, not only for SharePoint but for the whole Office platform were being built, Microsoft needed to provide developers with a common design language - one that would help them seamlessly integrate their solutions while remaining productive.
A while back Microsoft released the Office UI Fabric: a front-end toolkit that helps you leverage Office design language in your solutions for the Office platform. Office UI Fabric consists of common styling elements as well as a number of components used throughout the different Office-family products.
Office UI Fabric components - sample or production-ready?
To demonstrate how the Office UI Fabric components work, next to their styling, Microsoft built sample JavaScript implementations using jQuery. You can view the results at dev.office.com/fabric. And while it would make you think that the sample jQuery code is helpful, things look different when you start implementing them in your Angular application.
The sample component implementations are all based on jQuery, which doesn’t translate directly into Angular or any other framework for that matter. Every framework has its own architecture and its own way of dealing with component lifecycle, state and events. So while it’s a nice reference implementation, eventually you will end up rebuilding the components for your framework.
Angular directives for Office UI Fabric
The only way you should consider for using Office UI Fabric in your Angular applications
Angular is one of the most popular and widely adopted frameworks for developing web applications. When building Angular applications for the Office platform, you can easily benefit of the Office UI Fabric design language. But, as you might expect, it’s using the components that is the challenge. However it’s using the common components that is exactly what makes your applications look and feel like a part of the Office family.
Last year, fellow Office MVP, Andrew Connell saw these challenges too and started a community effort around implementing Office UI Fabric components as a set of Angular directives. Angular directives for Office UI Fabric, called ngOfficeUIFabric for short, is a fully community-driven, open source project driven by Andrew and team of community contributors towards a common goal of simplifying using the Office UI Fabric components in Angular applications. The project is run transparently on GitHub and Slack.
At the time of writing this article we are closing in on a full feature parity with the Office UI Fabric. The most commonly used components are already available as native Angular directives and if you are using Angular for building applications on the Office platform I would strongly recommend you take a look at ngOfficeUIFabric. Yes, it makes it easier for you to use Office UI Fabric in your application. But did you know that ngOfficeUIFabric actually offers more than the Office UI Fabric?
ngOfficeUIFabric - it’s more than UX
Besides making it easy to use Office UI Fabric in Angular applications, another important goal of the ngOfficeUIFabric initiative is to provide a set of fully functional components. Let me illustrate what we mean by that using the Table component as an example.
Table component in the Office UI Fabric
One of the components provided with the Office UI Fabric is Table.
The Table component is meant for rendering tabular data in Office applications. If you look at the sample implementation on the Office UI Fabric website, you see that it does exactly that. But looking at that table you might think of some other capabilities that you would expect of a data table.
Table directive in ngOfficeUIFabric
If you look at the way we implemented the Table component as a native Angular directive in ngOfficeUIFabric, you will see a fully functional component.
The markup you would use for this table in Angular is:
<uif-table>
<uif-table-row>
<uif-table-row-select></uif-table-row-select>
<uif-table-header>File name</uif-table-header>
<uif-table-header>Location</uif-table-header>
<uif-table-header>Modified</uif-table-header>
<uif-table-header>Type</uif-table-header>
</uif-table-row>
<uif-table-row ng-repeat="f in files">
<uif-table-row-select></uif-table-row-select>
<uif-table-cell></uif-table-cell>
<uif-table-cell></uif-table-cell>
<uif-table-cell></uif-table-cell>
<uif-table-cell></uif-table-cell>
</uif-table-row>
</uif-table>
At first it seems a lot like the table you see in the Office UI Fabric, but if you look closer you will see that the ngOfficeUIFabric Table directive actually offers you more.
You can see working demos of everything that I’m about to discuss on the ngOfficeUIFabric demo page at http://ngofficeuifabric.com/demos/uifTable/.
Marking rows as selected
When presenting data in a table you might want to highlight some rows. You can do that easily using the uif-selected
attribute on the <uif-table-row>
directive.
<uif-table>
<uif-table-row>
<uif-table-row-select></uif-table-row-select>
<uif-table-header>File name</uif-table-header>
<uif-table-header>Location</uif-table-header>
<uif-table-header>Modified</uif-table-header>
<uif-table-header>Type</uif-table-header>
</uif-table-row>
<uif-table-row ng-repeat="f in files" uif-selected="{{f.isSelected}}">
<uif-table-row-select></uif-table-row-select>
<uif-table-cell></uif-table-cell>
<uif-table-cell></uif-table-cell>
<uif-table-cell></uif-table-cell>
<uif-table-cell></uif-table-cell>
</uif-table-row>
</uif-table>
Selecting rows
Another thing that you might want to do, when presenting data in a table, is to allow users to interact with the data. By selecting rows they could either perform operations on them, like editing or deleting them, or could load additional details about them.
You can configure the ngOfficeUIFabric Table to allow selecting one or more rows. The row selection mode is controlled using the uif-row-select-mode
attribute on the <uif-table>
directive.
To allow selecting a single row, you would set the uif-row-select-mode
attribute to single
:
<uif-table uif-row-select-mode="single">
<uif-table-row>
<uif-table-row-select></uif-table-row-select>
<uif-table-header uif-order-by="fileName">File name</uif-table-header>
<uif-table-header>Location</uif-table-header>
<uif-table-header uif-order-by="modified">Modified</uif-table-header>
<uif-table-header uif-order-by="type">Type</uif-table-header>
<uif-table-header uif-order-by="size">Size</uif-table-header>
</uif-table-row>
<uif-table-row ng-repeat="f in files" uif-item="f">
<uif-table-row-select></uif-table-row-select>
<uif-table-cell></uif-table-cell>
<uif-table-cell></uif-table-cell>
<uif-table-cell></uif-table-cell>
<uif-table-cell></uif-table-cell>
<uif-table-cell></uif-table-cell>
</uif-table-row>
</uif-table>
The important part here is, that in conjunction with
uif-row-select-mode="single"
, you also need to pass the data item to the directive using theuif-item
attribute on the<uif-table-row>
directive. That way you will be able to retrieve the selected data item (not table row!) later.
Similarly, you can allow for selecting multiple items in the table by setting the uif-row-select-mode
attribute to multiple
:
<uif-table uif-row-select-mode="multiple">
<uif-table-row>
<uif-table-row-select></uif-table-row-select>
<uif-table-header uif-order-by="fileName">File name</uif-table-header>
<uif-table-header>Location</uif-table-header>
<uif-table-header uif-order-by="modified">Modified</uif-table-header>
<uif-table-header uif-order-by="type">Type</uif-table-header>
<uif-table-header uif-order-by="size">Size</uif-table-header>
</uif-table-row>
<uif-table-row ng-repeat="f in files" uif-item="f">
<uif-table-row-select></uif-table-row-select>
<uif-table-cell></uif-table-cell>
<uif-table-cell></uif-table-cell>
<uif-table-cell></uif-table-cell>
<uif-table-cell></uif-table-cell>
<uif-table-cell></uif-table-cell>
</uif-table-row>
</uif-table>
Another gem, that you might not know about when using the multiple row selection mode in the ngOfficeUIFabric Table, is that using the checkbox in the top left corner of the table you can easily toggle selection of all rows!
Sorting table data
The ngOfficeUIFabric Table directive supports rendering its rows using the ngRepeat directive. With that, you could easily have your data rendered using ordering of your choice by using the standard Angular orderBy
filter. But did you know that the ngOfficeUIFabric Table directive also supports live sorting?
In order to enable sorting in your table rendered using the ngOfficeUIFabric Table directive, you have to do two things.
First, for each column, that should be allowed to sort by, add the uif-order-by
attribute to the corresponding <uif-table-header>
directive. The value of that attribute should be set to the corresponding property of your data item that should be used for sorting:
<uif-table>
<uif-table-row>
<uif-table-row-select></uif-table-row-select>
<uif-table-header uif-order-by="fileName">File name</uif-table-header>
<uif-table-header>Location</uif-table-header>
<uif-table-header uif-order-by="modified">Modified</uif-table-header>
<uif-table-header uif-order-by="type">Type</uif-table-header>
<uif-table-header uif-order-by="size">Size</uif-table-header>
</uif-table-row>
Notice how the Location column doesn’t contain the
uif-order-by
attribute and therefore won’t enabled for sorting.
Then, extend the ngRepeat directive for your table row definition with the standard Angular orderBy filter hooking it up to the table sorting controls:
<uif-table-row ng-repeat="f in files | orderBy:table.orderBy:!table.orderAsc">
...
</uif-table-row>
table.orderBy
and table.orderAsc
are fixed names automatically added to the current scope by the Table directive.
With such setup you can now sort your table data directly on the page:
Summary
If you’re building Angular applications on the Office platform, you might have heard about the Angular directives for Office UI Fabric (ngOfficeUIFabric for short). ngOfficeUIFabric offers native Angular directives for the different Office UI Fabric components. The great thing is that these directives not only make it easier to use the Office UI Fabric in your Angular applications but also offer additional functionality that your users will benefit of. I would strongly encourage you to take a look at the live demos of the different directives at http://ngofficeuifabric.com/.