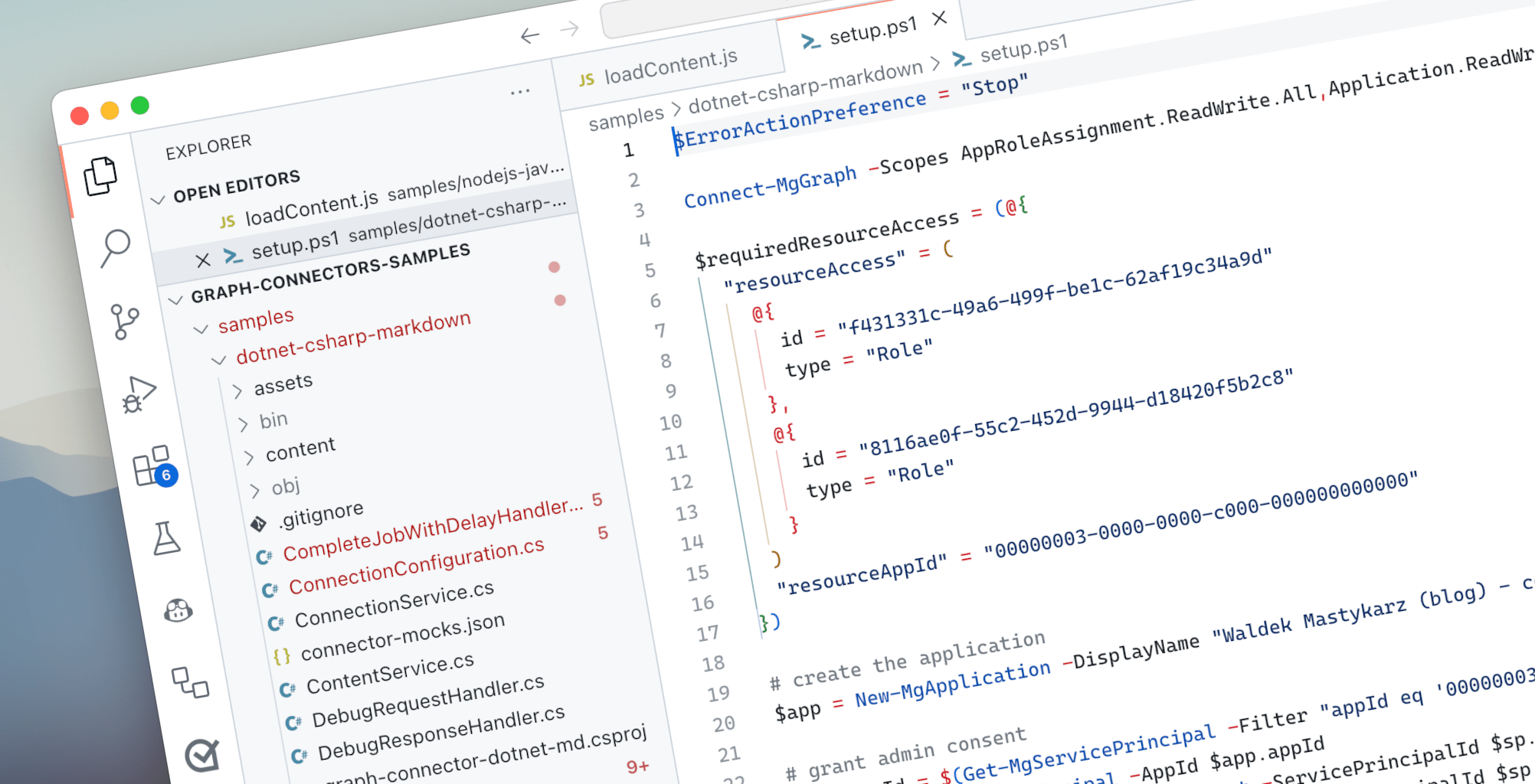
Create an Entra app with API permissions, admin consent and a secret using Microsoft Graph PowerShell SDK
When building apps for Microsoft 365, the first step is to create an Entra app registration. Here’s how to do it using Microsoft Graph PowerShell SDK.
First things first: Entra app registrations
In my previous article, I told you about the necessity of having an Entra app registration when building apps for Microsoft 365. I also showed you, how to script creating and configuring an Entra app registration using the CLI for Microsoft 365. If you’re using PowerShell you might be more inclined to use the Microsoft Graph PowerShell SDK instead, and here’s how you can write a similar script using it.
What’s Microsoft Graph PowerShell SDK
Microsoft Graph PowerShell SDK is a set of cmdlets that wrap Microsoft Graph APIs and expose them in PowerShell. Because the SDK manages auth for you, it’s a very convenient way of using Microsoft Graph APIs for writing automation scripts.
Create Entra app registration using Microsoft Graph PowerShell SDK
Here’s a PowerShell script that creates an Entra app registration for use with a Microsoft Graph connector. The script configures API permissions, grants admin consent, creates a secret, and stores the information in .NET user secrets for use with your application.
Let’s go through it step by step.
Connect-MgGraph -Scopes AppRoleAssignment.ReadWrite.All,Application.ReadWrite.All -NoWelcome
$requiredResourceAccess = (@{
"resourceAccess" = (
@{
id = "f431331c-49a6-499f-be1c-62af19c34a9d"
type = "Role"
},
@{
id = "8116ae0f-55c2-452d-9944-d18420f5b2c8"
type = "Role"
}
)
"resourceAppId" = "00000003-0000-0000-c000-000000000000"
})
# create the application
$app = New-MgApplication -DisplayName "Waldek Mastykarz (blog) - connector (.net)" -RequiredResourceAccess $requiredResourceAccess
# grant admin consent
$graphSpId = $(Get-MgServicePrincipal -Filter "appId eq '00000003-0000-0000-c000-000000000000'").Id
$sp = New-MgServicePrincipal -AppId $app.appId
New-MgServicePrincipalAppRoleAssignment -ServicePrincipalId $sp.Id -PrincipalId $sp.Id -AppRoleId "f431331c-49a6-499f-be1c-62af19c34a9d" -ResourceId $graphSpId
New-MgServicePrincipalAppRoleAssignment -ServicePrincipalId $sp.Id -PrincipalId $sp.Id -AppRoleId "8116ae0f-55c2-452d-9944-d18420f5b2c8" -ResourceId $graphSpId
# create client secret
$cred = Add-MgApplicationPassword -ApplicationId $app.id
# store values in user secrets
dotnet user-secrets init
dotnet user-secrets set "AzureAd:ClientId" $app.appId
dotnet user-secrets set "AzureAd:ClientSecret" $cred.secretText
dotnet user-secrets set "AzureAd:TenantId" $($(Get-MgContext).TenantId)
Sign in with Microsoft Graph PowerShell SDK
Before you can use Microsoft Graph PowerShell SDK, you need to log in with your work or school account. You do this using the Connect-MgGraph
cmdlet. While invoking it, you need to specify the list of scopes that you’ll need for your script.
TIP: To find out if you’re using the minimal permissions for your app, use the Microsoft 365 Developer Proxy.
Create Entra app registration
After signing in with Microsoft Graph PowerShell SDK, we use the New-MgApplication
cmdlet to create a new Entra app registration. Along with the name, we use the RequiredResourceAccess
argument to specify the list of API permissions to grant the application. This definition seems a bit cryptic, and while you could extend the script to look it up based on the scope names, another way to get it, is to configure the Entra app registration manually in the Azure Portal and get the API permission information from the app’s manifest.
Grant admin consent
Next, we’ll grant admin consent for API permissions that we’ve just set on our app. To do this, we first need the object ID of the Microsoft Graph service principal. We retrieve it, using the Get-MgServicePrincipal
cmdlet filtering by the well-known Microsoft Graph app ID.
Then, we create a new service principal for our Entra app registration. We do this using the New-MgServicePrincipal
cmdlet passing the app ID of our newly created Entra app registration.
With these prerequisites in place, we’re ready to grant admin consent for the API permissions for our app. For each permission, we invoke the New-MgServicePrincipalAppRoleAssignment
cmdlet passing the relevant information through args: both ServicePrincipalId
and PrincipalId
point to the object ID of the service principal of our Entra app registration, ResourceId
references the object ID of the Microsoft Graph service principal and AppRoleId
points to the application permissions for which we want to grant consent.
Create secret
The next step is to create a client secret to make it possible to authenticate with application permissions. We do this using the Add-MgApplicationPassword
cmdlet, passing the object ID of our Entra app registration.
Store application information for use with the application
If you build an app for Microsoft 365 using .NET, you can use the dotnet user-secret
command to securely store secrets for use with your app. Start with initiating a secret store for your app by calling dotnet user-secret init
. Then, for each secret to store, use the dotnet user-secret set
command passing the name and the value of the secret.
Since the Entra app registration that we created only accepts authentication from accounts on the same tenant, we also need to store the tenant ID which we’ll need in our app for Microsoft 365. We can get it using the Get-MgContext
cmdlet.
To see this script in action, check out this sample Microsoft Graph connector that uses it.