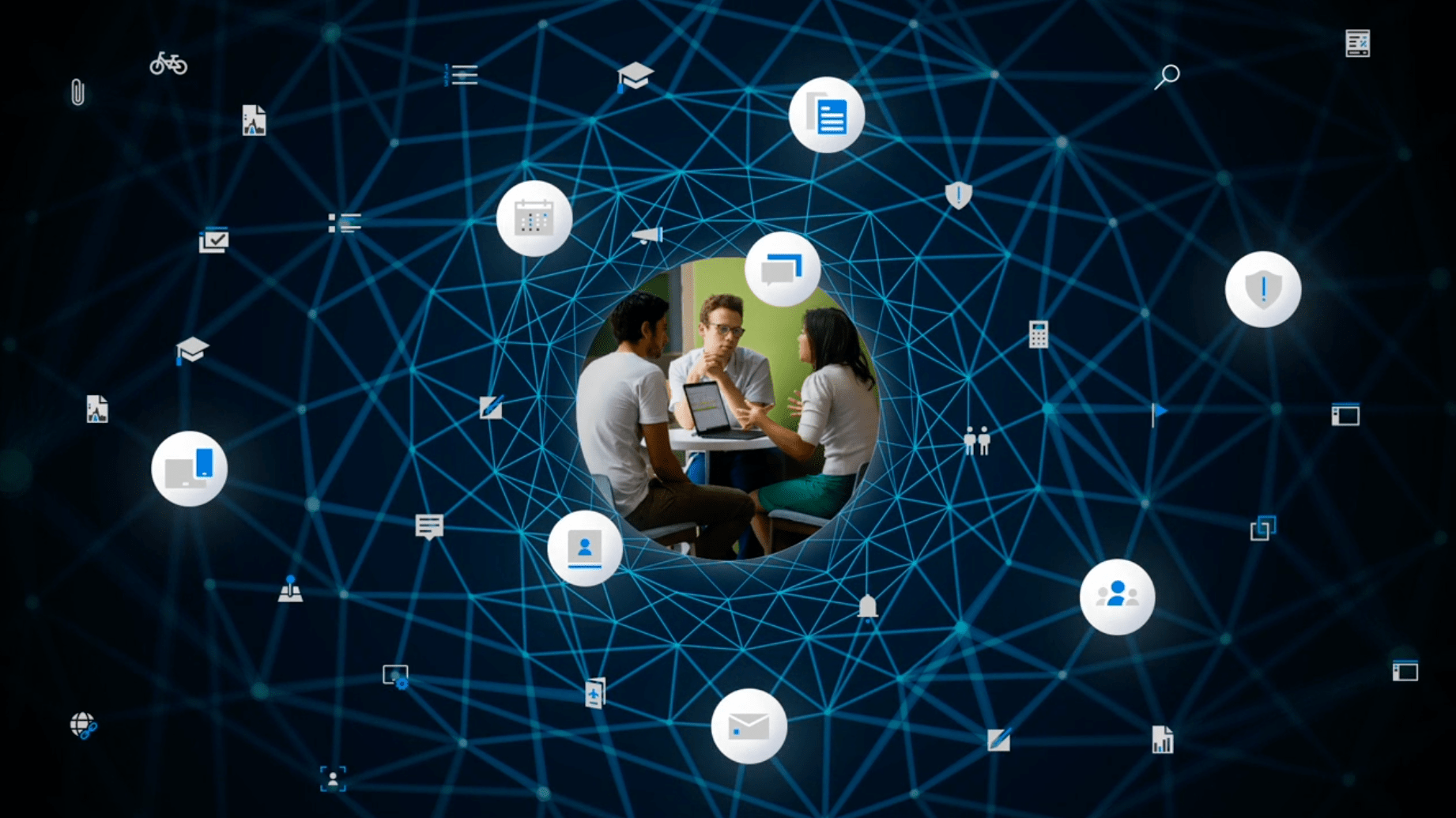
Quick tip: download user or group profile picture using Microsoft Graph JS SDK
When building your application on Microsoft 365 you might need to download the profile picture of a user or group. Here is how to do it using the Microsoft Graph SDK.
Microsoft Graph - The API to connect to Microsoft 365
Microsoft 365 consists of many different applications and is used by organizations of all sizes and in all markets. In total, there are 250 million users all over the world working with Microsoft 365 every day, creating files, sending emails, meeting, reading information stored in Microsoft 365 and more. All this information, bearing the necessary permissions, is available for you to use in your applications. And the way you do it is through Microsoft Graph: the API to connect to Microsoft 365.
Microsoft Graph not only allows you to connect your application to Microsoft 365 and tap into the insights stored in Microsoft 365. It also abstracts away the differences across the different products and services, giving you a consistent experience no matter what kind of information stored in Microsoft 365 you’re interested in.
The easiest way to use Microsoft Graph
The easiest way to use Microsoft Graph in your application is through an SDK. Graph SDKs offer you design-time type safety, allowing you to catch errors as you type rather than finding out about them on runtime. What’s more, they abstract away implementation of the necessary plumbing such as authentication or handling throttling, allowing you to focus on building your application.
There are Graph SDKs available for the most popular programming languages and platforms and they’re being continuously updated, giving you access to all of Graph endpoints in your applications.
Download user or group profile picture using Microsoft Graph JS SDK
When building your application on Microsoft 365, you might want to display the profile picture of a user or a group. If you use the Microsoft Graph JavaScript SDK, you’d do it by issuing the following request:
const url = window.URL || window.webkitURL;
try {
const response = await graphClient
.api(`/groups/${group.id}/photo/$value`)
.responseType(ResponseType.RAW)
.get();
if (!response.ok) {
throw response.statusText;
}
const blob = await response.blob();
const imageUrl = url.createObjectURL(blob);
}
catch (e) {
console.error(e);
}
When downloading profile picture from Microsoft Graph, whether it’s a user or a group picture, you need to explicitly state that you want to retrieve the picture itself rather than its metadata ($value
at the end of the API call):
const response = await graphClient
.api(`/groups/${group.id}/photo/$value`)
Then, before executing the request, you also need to instruct the SDK to retrieve raw data from Microsoft Graph:
const response = await graphClient
.api(`/groups/${group.id}/photo/$value`)
.responseType(ResponseType.RAW)
If the request succeeded, you need to take the blob and turn it into an object URL, which you can then use with an img
tag or in CSS:
const url = window.URL || window.webkitURL;
// [...]
const blob = await response.blob();
const imageUrl = url.createObjectURL(blob);
If the user or a group doesn’t have a profile picture configured, the request will fail, which you need to handle as well to avoid your application from crashing.
const response = await graphClient
.api(`/groups/${group.id}/photo/$value`)
.responseType(ResponseType.RAW)
.get();
if (!response.ok) {
// there was an error retrieving the picture
throw response.statusText;
}
That’s it! If you want to know more about building applications for Microsoft 365 using Microsoft Graph, check out the getting started page.