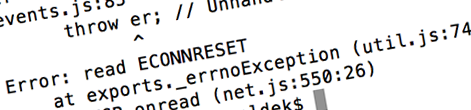
ECONNRESET error when connecting to Office 365 from node.js
When trying to connect to Office 365 from your node.js application you get the ECONNRESET error.
Your apps on your terms
The new Office 365 APIs empower us to build powerful solutions leveraging the capabilities of Office 365 using virtually any technology. Microsoft has been heavily investing to provide us with a variety of SDKs for different platforms other than .NET to help us interact with Office 365.
Office 365 APIs and Azure AD authentication flow are all modern APIs exposed as REST services. This means, that even if there is no SDK for your platform yet, you can still build an app using your technology. Once you understand the Azure AD authentication flow you will connect your app to Office 365 interacting with the data stored across your tenant.
Building Office 365 API apps with node.js
Node.js is a powerful platform for building scalable network applications. In a world where developing in JavaScript becomes more and more common, building node.js is very appealing because it allows you to leverage your existing skills and be productive quickly.
Node.js is one of those platforms that don’t have an Office 365 APIs SDK yet and if you choose to build a node.js app to connect to Office 365 using the Office 365 APIs you will need to rely solely on the REST endpoints manually issuing and processing web requests.
Issuing a web request to Office 365 APIs
Assuming you have successfully authenticated and authorized with Azure AD, you would issue a web request to one of the Office 365 APIs. That request might look like:
var https = require("https"),
accessToken = 'ABC...';
https.get({
hostname: "contoso.sharepoint.com",
path: "/_api/search/query?queryText='size>0'",
headers: {
"Authorization": "Bearer " + accessToken,
"Accept": "application/json;odata=verbose"
}
}, function(res) {});
Unfortunately if you execute this request, your node.js app will work for a moment and then fail with the ECONNRESET error.
Inconvenient node.js and executing web requests to Office 365
It turns out that the error is caused by the SSL certificate used on Office 365. Node.js seems to have problems establishing the handshake and as a result the connection fails. To solve the issue you have to include the following lines in each web request to Office 365 to tell node.js how to handle the SSL certificate used with Office 365:
secureOptions: require('constants').SSL_OP_NO_TLSv1_2,
ciphers: 'ECDHE-RSA-AES256-SHA:AES256-SHA:RC4-SHA:RC4:HIGH:!MD5:!aNULL:!EDH:!AESGCM',
honorCipherOrder: true
After changing your web request to:
var https = require("https"),
accessToken = 'ABC...';
https.get({
hostname: "contoso.sharepoint.com",
path: "/_api/search/query?queryText='size>0'",
headers: {
"Authorization": "Bearer " + accessToken,
"Accept": "application/json;odata=verbose"
},
secureOptions: require('constants').SSL_OP_NO_TLSv1_2,
ciphers: 'ECDHE-RSA-AES256-SHA:AES256-SHA:RC4-SHA:RC4:HIGH:!MD5:!aNULL:!EDH:!AESGCM',
honorCipherOrder: true
}, function(res) {});
your request to Office 365 will succeed and you will be able to proceed with processing the response data.