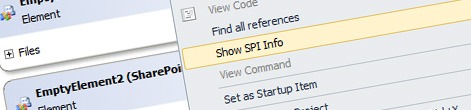
Extending all SharePoint Project Item Types – Extending Visual Studio SharePoint Developer Tools tip #10
When extending standard functionality of SharePoint Project Item Types in Visual Studio 2010 SharePoint Developer Tools there are scenarios when you might want your extensions to be available on all types of SharePoint Project Items. Find out how to make your custom functionality available to all SharePoint Project Item Types.
Creating custom SPI Types extensions 101 and why it’s challenging
Imagine the following scenario: you’re working on a new extension for the Visual Studio 2010 SharePoint Developer Tools that should work in the context of any SharePoint Project Item (SPI) Type. If you have worked with the extensibility framework of the new developer tools, you know that in order to create an extension for a SPI Type you have to create a custom class that implements the ISharePointProjectItemTypeExtension interface and is decorated with the ExportAttribute and the SharePointProjectItemTypeAttribute attributes. According to the SDK both attributes are required for the extension to be discovered by the project system. The challenge with this approach is that it binds the extension to a specific SPI Type. In order to make your extension available to all SPI Types you could of course decorate your extension with multiple SharePointProjectItemTypeAttribute attributes, but anytime a new SPI Type would appear on the market you would have to modify your extension to support the new type as well. Not really future-proof is it? So how do you register your custom extension with all SPI Types including those that haven’t been created yet?
Getting things done
In order to create a custom extension registered with all SPI Types you have to create not a SharePoint Project Item Type Extension but a SharePoint Project Extension instead! Using the extensibility API of the Visual Studio 2010 SharePoint Developer Tools, you can iterate through all registered SPI Types and register your extension with them.
To illustrate this case a little more let’s create a new extension that will display the name and the type of the selected SPI in a dialog window.
Let’s start with creating a new VSIX project that will contain the extension. Let’s call it GlobalSPITypeExtension.
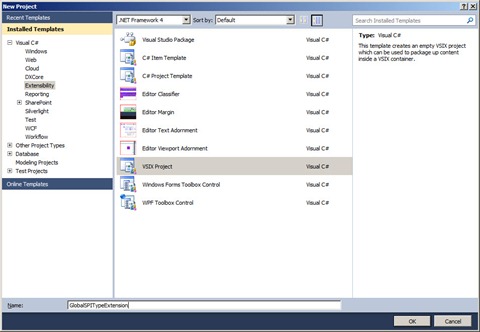
Next let’s add references to assemblies that we will need in order to create the extension:
- Microsoft.VisualStudio.SharePoint
- System
- System.ComponentModel.Composition
- System.Windows.Forms
You can also unload the project and paste the following code snippet to get those references in place:
<ItemGroup>
<Reference Include="Microsoft.VisualStudio.SharePoint, Version=10.0.0.0, Culture=neutral, PublicKeyToken=b03f5f7f11d50a3a, processorArchitecture=MSIL" />
<Reference Include="System" />
<Reference Include="System.ComponentModel.Composition" />
<Reference Include="System.Windows.Forms" />
</ItemGroup>
With that in place let’s add a new class called SPIInfoProjectExtension:
[Export(typeof(ISharePointProjectExtension))]
public class SPIInfoProjectExtension : ISharePointProjectExtension
{
public void Initialize(ISharePointProjectService projectService)
{
}
}
The next part is to register the extension with all available SPI Types:
[Export(typeof(ISharePointProjectExtension))]
public class SPIInfoProjectExtension : ISharePointProjectExtension
{
public void Initialize(ISharePointProjectService projectService)
{
foreach (ISharePointProjectItemType spit in projectService.ProjectItemTypes.Values)
{
spit.ProjectItemMenuItemsRequested += new EventHandler<SharePointProjectItemMenuItemsRequestedEventArgs>(spit_ProjectItemMenuItemsRequested);
}
}
void spit_ProjectItemMenuItemsRequested(object sender, SharePointProjectItemMenuItemsRequestedEventArgs e)
{
IMenuItem showSPIInfoMenuItem = e.ViewMenuItems.Add("Show SPI Info");
showSPIInfoMenuItem.Click += new EventHandler<MenuItemEventArgs>(showSPIInfoMenuItem_Click);
}
void showSPIInfoMenuItem_Click(object sender, MenuItemEventArgs e)
{
}
}
As mentioned before, in this sample we will add a new menu to the SPI context menu that will display the SPI name and type in a dialog window.
The last step is to implement the logic of retrieving and displaying the information about the selected SPI:
[Export(typeof(ISharePointProjectExtension))]
public class SPIInfoProjectExtension : ISharePointProjectExtension
{
public void Initialize(ISharePointProjectService projectService)
{
foreach (ISharePointProjectItemType spit in projectService.ProjectItemTypes.Values)
{
spit.ProjectItemMenuItemsRequested += new EventHandler<SharePointProjectItemMenuItemsRequestedEventArgs>(spit_ProjectItemMenuItemsRequested);
}
}
void spit_ProjectItemMenuItemsRequested(object sender, SharePointProjectItemMenuItemsRequestedEventArgs e)
{
IMenuItem showSPIInfoMenuItem = e.ViewMenuItems.Add("Show SPI Info");
showSPIInfoMenuItem.Click += new EventHandler<MenuItemEventArgs>(showSPIInfoMenuItem_Click);
}
void showSPIInfoMenuItem_Click(object sender, MenuItemEventArgs e)
{
ISharePointProjectItem spi = e.Owner as ISharePointProjectItem;
if (spi != null)
{
MessageBox.Show(String.Format("SPI Name: '{0}', Type: '{1}'", spi.Name, spi.ProjectItemType.Name));
}
}
}
If you press F5, the extension will be built and the Experimental Instance of Visual Studio 2010 will start with the extension automatically loaded. When you click right mouse button on a SPI in your project, a new menu item will be visible in the context menu:
Once you click it, the information about the SPI will be displayed:
Even if you choose a SPI of another type, you will still be able to view the information of the selected item:
Summary
Visual Studio 2010 SharePoint Developer Tools are highly extensible what allows developers to tailor their toolkit to their specific needs. Among many different extensibility points, developers are able to extend the standard functionality of SharePoint Project Item Types. Although by default SharePoint Project Item Types extensions can be registered with specific types only, you can create a custom extension that is registered with all available SPI Types by creating a SharePoint Project Extension rather than a SharePoint Project Item Type Extension.
Technorati Tags: Visual Studio 2010,SharePoint 2010