Extending Visual Studio SharePoint development tools with custom Project menu options
SharePoint 2010 ships with a great development story. Using the new Visual Studio SharePoint development tools you can create SharePoint solutions easier and faster. Visual Studio SharePoint development tools provide you out of the box with a great number of Project and Item Templates which allow you to create the most commonly used SharePoint artifacts. Using the new SharePoint Server Explorer you can explore all the different objects within your Site Collection and using the Validation Rules and Deployment Steps you can package your work into a WSP package and deploy it to your server with a single mouse click. Yet I think that the most exciting part about the new Visual Studio SharePoint development tools is the extensibility, because the possibilities are almost limitless.
Extending Visual Studio SharePoint development tools
Visual Studio SharePoint development tools are built using the Managed Extensibility Framework also known as MEF. MEF is a framework that makes creating extensible applications easier. The new SharePoint development tools fully leverage the power of MEF allowing you to extend almost every single part of the tools. If you’re interested in the extensibility possibilities of the Visual Studio SharePoint development tools there is a great news for you. In spite of the fact that the Visual Studio 2010 Beta 2 has been releases only a few weeks ago, there is a lot of great content on MSDN about all the new functionality including some samples of how you could extend the SharePoint Tools.
Adding custom Project menu options to a SharePoint project in Visual Studio SharePoint development tools
One of the things that you might want to extend in the Visual Studio SharePoint development tools are the menu items in the context menu item of a SharePoint project. Out of the box the following menu options are available: Deploy, Clean, Package and Retract.
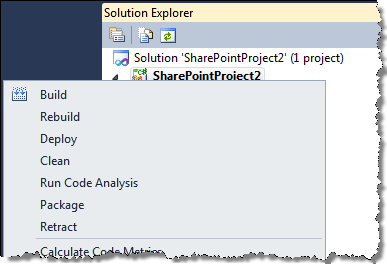
While these should be sufficient in the most scenarios, imagine that your team has some custom code which could be executed in the context of a SharePoint Project, like for example a Quick Deploy task to copy some files. You could of course create a custom Deployment Configuration and hook the code up there, but switching the Active Deployment Configuration every time you want to do a Quick Deploy doesn’t sound very efficient, does it? A custom SharePoint Project menu option seems like a better idea.
Adding custom menu options to the SharePoint Project menu is really straight forward. You start off with creating a VSIX project, just like you would do for every other Visual Studio SharePoint development tools extension.
After you configured the VSIX package, you add a new SharePointProjectExtension to your Implementation project:
[Export(typeof(ISharePointProjectExtension))]
public class CustomProjectMenuOptionExtension : ISharePointProjectExtension
{
public void Initialize(ISharePointProjectService projectService)
{
}
}
The next step is to add your custom menu item. This can be done by adding an event handler to the ISharePointProjectService.ProjectMenuItemsRequested event which fires every time the user clicks with the right mouse button on a SharePoint Project:
[Export(typeof(ISharePointProjectExtension))]
public class CustomProjectMenuOptionExtension : ISharePointProjectExtension
{
public void Initialize(ISharePointProjectService projectService)
{
projectService.ProjectMenuItemsRequested += new EventHandler<SharePointProjectMenuItemsRequestedEventArgs>(projectService_ProjectMenuItemsRequested);
}
void projectService_ProjectMenuItemsRequested(object sender, SharePointProjectMenuItemsRequestedEventArgs e)
{
}
}
The Visual Studio SharePoint development tools API allows you to add a custom menu option in two places: in the Action section or the Add section:
Adding a new menu items is very simple and can be done using the IMenuItemCollection.Add() method:
void projectService_ProjectMenuItemsRequested(object sender, SharePointProjectMenuItemsRequestedEventArgs e)
{
IMenuItem customMenuItem = e.ActionMenuItems.Add("Hello World Action");
IMenuItem customAddMenuItem = e.AddMenuItems.Add("Hello World Add");
}
The last thing left is to hook up your custom code that will be executed once the menu item has been clicked on. You can do this by adding an event handler to the IMenuItem.Click event:
void projectService_ProjectMenuItemsRequested(object sender, SharePointProjectMenuItemsRequestedEventArgs e)
{
IMenuItem customActionMenuItem = e.ActionMenuItems.Add("Hello World Action");
IMenuItem customAddMenuItem = e.AddMenuItems.Add("Hello World Add");
customActionMenuItem.Click += new EventHandler<MenuItemEventArgs>(customActionMenuItem_Click);
}
void customActionMenuItem_Click(object sender, MenuItemEventArgs e)
{
System.Windows.Forms.MessageBox.Show("Hello World from custom menu Action");
}
And that’s really all that you need to do to add your custom menu items to SharePoint Projects!
One more cool feature that the Visual Studio SharePoint development tools API ships with is the ability to allow you to conditionally enable menu items. Imagine that you created a Quick Deploy menu item. Quick deployments are in general not supported by Sandbox Solutions so there is no point of making the menu item available if the SharePoint Project is a Sandbox Solution. In order to disable the menu item, you can set the value of the IMenuItem.IsEnabled property to false:
void projectService_ProjectMenuItemsRequested(object sender, SharePointProjectMenuItemsRequestedEventArgs e)
{
IMenuItem customActionMenuItem = e.ActionMenuItems.Add("Hello World Action");
IMenuItem customAddMenuItem = e.AddMenuItems.Add("Hello World Add");
customActionMenuItem.Click += new EventHandler<MenuItemEventArgs>(customActionMenuItem_Click);
customActionMenuItem.IsEnabled = e.Project.IsSandboxedSolution == false;
}
If you now create a new SharePoint Project targeted to the Sandbox you will see, that the Hello World Action menu item is disabled:
Summary
Visual Studio SharePoint development tools ship with a great number of components that make development on the SharePoint platform easier and more efficient. Next to that they provide a great framework for extending the tools with custom commands and templates. By leveraging the extensibility API every SharePoint development team is able to tailor the Visual Studio SharePoint development tools to their specific needs.
Download: Example solution for extending SharePoint Project menu with custom options (46KB, ZIP)
Technorati Tags: SharePoint 2010,Visual Studio 2010