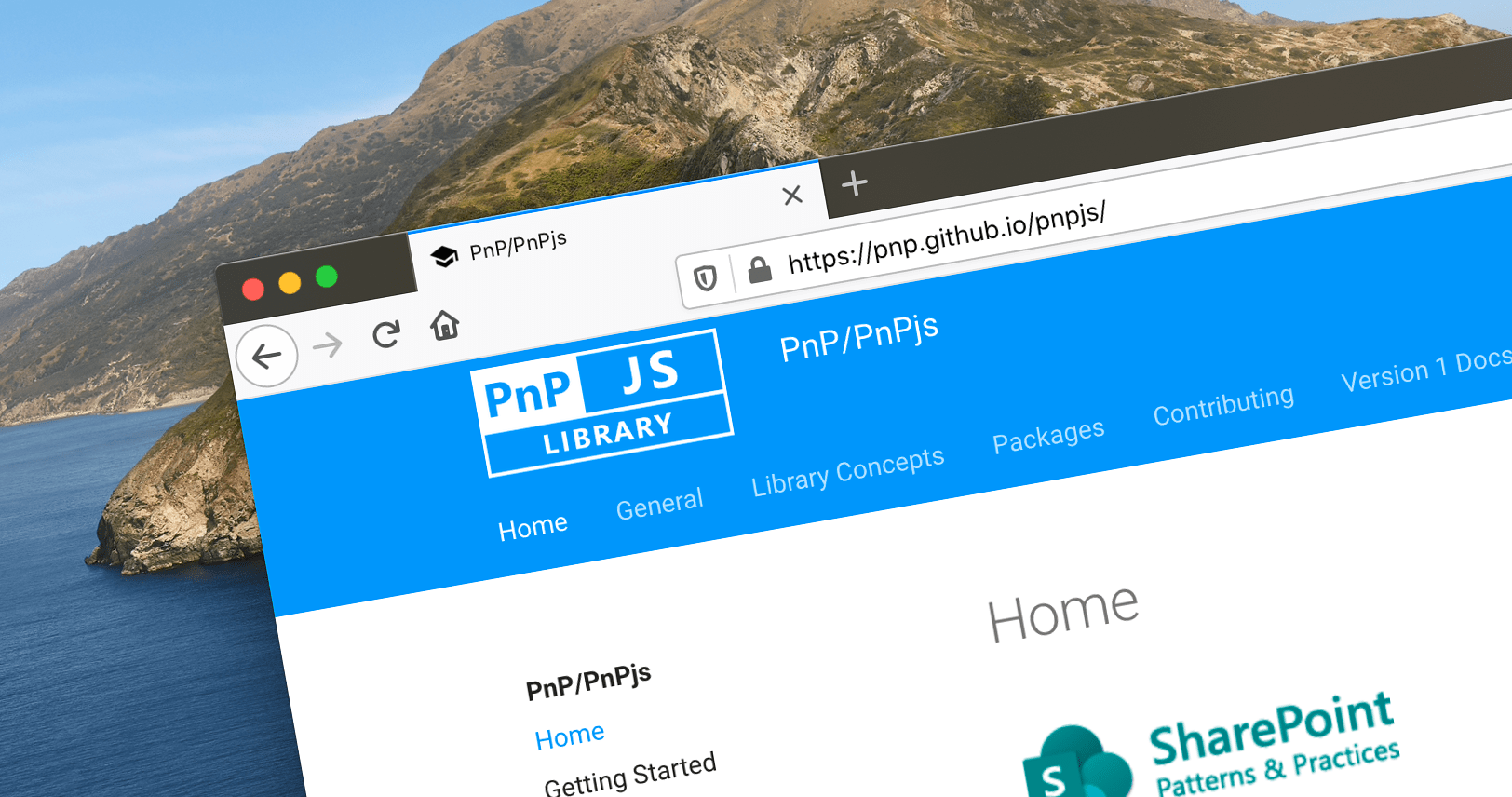
#12 If you don’t use PnPjs you miss out
Do you prefer this:
this._httpClient.get(this._organizationListUrl +
"/fields?select=ID,InternalName,Title,FieldTypeKind," +
"Choices,RichText,AllowMultipleValues,MaxLength,LookupList&" +
"$fitler=Hidden eq false and FieldTypeKind ne 12 " +
"and InternalName ne 'AppEditor' and InternalName ne 'AppAuthor'",
SPHttpClient.configurations.v1, this._getStandardHttpClientOptions())
.then((response: SPHttpClientResponse) => {
return response.json();
})
.then((json: { value: ISPField[] }) => {
// ...
});
Or this:
sp.web
.lists.getByTitle('Organizations')
.fields
.filter("Hidden eq false and FieldTypeKind ne 12 and " +
"InternalName ne 'AppEditor' and InternalName ne 'AppAuthor'")
.select('ID', 'InternalName', 'Title', 'FieldTypeKind',
'Choices', 'RichText', 'AllowMultipleValues', 'MaxLength', 'LookupList')
.get()
.then((fields: IFieldInfo[]): void => {
// ...
});
How about this:
public static createList(context: IWebPartContext,
listTitle: string,
listDescription: string,
baseTemplate: number,
enableApproval: boolean = true,
enableVersioning: boolean = false): Promise {
console.log(`create list ${listTitle}`);
const reqJSON: any = JSON.parse(
`{
"@odata.type": "#SP.List",
"AllowContentTypes": true,
"BaseTemplate": ${baseTemplate},
"ContentTypesEnabled": true,
"Description": "${listDescription}",
"Title": "${listTitle}"
}`);
if (enableApproval){
reqJSON.EnableModeration = true;
}
if (enableVersioning){
reqJSON.EnableVersioning = true;
}
return context.spHttpClient.post(context.pageContext.web.absoluteUrl +
"/_api/web/lists",
SPHttpClient.configurations.v1,
{
body: JSON.stringify(reqJSON),
headers: {
"accept": "application/json",
"content-type": "application/json"
}
})
.then((response: SPHttpClientResponse): Promise => {
console.log("result: " + response.status);
return response.json();
});
}
Or this:
public static createList(listTitle: string,
listDescription: string,
baseTemplate: number,
enableApproval: boolean = true,
enableVersioning: boolean = false): Promise {
console.log(`create list ${listTitle}`);
return sp.web
.lists.add(listTitle, listDescription, baseTemplate, true, {
EnableModeration: enableApproval,
EnableVersioning: enableVersioning
})
.then(listResponse => listResponse.data);
}
PnPjs simplifies communicating with SharePoint allowing you to focus on what truly matters. Look again at the examples above, which code is easier to read? And I bet you haven’t even noticed the typos that would break on runtime.
Recently I’ve been refactoring a couple of projects from REST to PnPjs. I gotta tell you: nothing beats intellisense and design-time checks. If you don’t want to do it for the fluent syntax then do it for your team or for your own sanity. I promise you won’t be disappointed.
Get started with PnPjs at https://pnp.github.io/pnpjs/.
Looking forward to hear about your next refactoring project.
Others found also helpful: