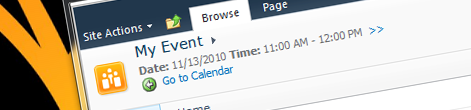
Inconvenient programmatically uploading files to the Document Library in Meeting Workspace
There are scenarios when you might to programmatically copy or upload a file to the Document Library in a Meeting Workspace. And while it doesn’t seem like rocket science at the first glance, there is one detail to keep in mind.
The anatomy of a Meeting Workspace
Meeting Workspace looks at the first glance like yet another Site Definition. It has an ONet file and a few configurations and depending on the selected flavor a few lists are being provisioned alongside with the site. If you analyze the Site Definition all you will see are out of the box Site Definitions without any extra fluff.
So what’s wrong with Meeting Workspaces?
Recently I’ve been asked to provide a solution for programmatically copying files to the Document Library in a Meeting Workspace. Because the Meeting Workspace is a separate site you cannot use the SPFile.CopyTo method. Instead you could use the following snippet:
SPSite site = SPContext.Current.Site;
SPWeb web = SPContext.Current.Web;
SPFile file = web.GetFile("/Shared%20Documents/Document.docx");
using (SPWeb meetingWorkspace = site.OpenWeb("My Event"))
{
SPList documentLibrary = meetingWorkspace.Lists["Document Library"];
documentLibrary.RootFolder.Files.Add(file.Name, file.OpenBinary());
}
Although the code works, if you navigate to the Meeting Workspace, the Document Library will say that there are no items to show in the view.
So has the file been copied or not? If you examine the value of the SPList.RootFolder.Files.Count property you will see that the file is there indeed and you can retrieve it as expected. So why does the Document Library keep saying that there are no items to display?
The anatomy of a Meeting Workspace revisited
When associating a new Calendar Item with a Workspace you have the choice to either create a new Meeting Workspace or associate the item with an existing one. And this is exactly when things get tricky.
By default you can associate a Meeting Workspace with multiple Calendar Items. Every time you associate a Meeting Workspace with a Calendar Item, a new instance is being registered with the Meeting Workspace. In order to separate files that belong to different instances, SharePoint uploads them to separate folders. The confusing thing is, that although the instance folders are not being displayed in the UI, they are there.
So, getting back to our code snippet for programmatically copying documents, in order to upload a document to the Document Library in a Meeting Workspace and make it display correctly with the right instance, you have to change the snippet as follows:
SPSite site = SPContext.Current.Site;
SPWeb web = SPContext.Current.Web;
string instanceId = "1";
SPFile file = web.GetFile("/Shared%20Documents/Document.docx");
using (SPWeb meetingWorkspace = site.OpenWeb("My Event"))
{
SPList documentLibrary = meetingWorkspace.Lists["Document Library"];
SPFolder instanceFolder = documentLibrary.RootFolder.SubFolders[instanceId];
instanceFolder.Files.Add(file.Name, file.OpenBinary());
}
If you go to the Document Library now, the document should appear in the view as expected.
One more important thing to notice is, that although I’ve used SharePoint 2010 as sample for this article, exactly the same approach can be used with SharePoint 2007.
Technorati Tags: SharePoint,SharePoint 2010