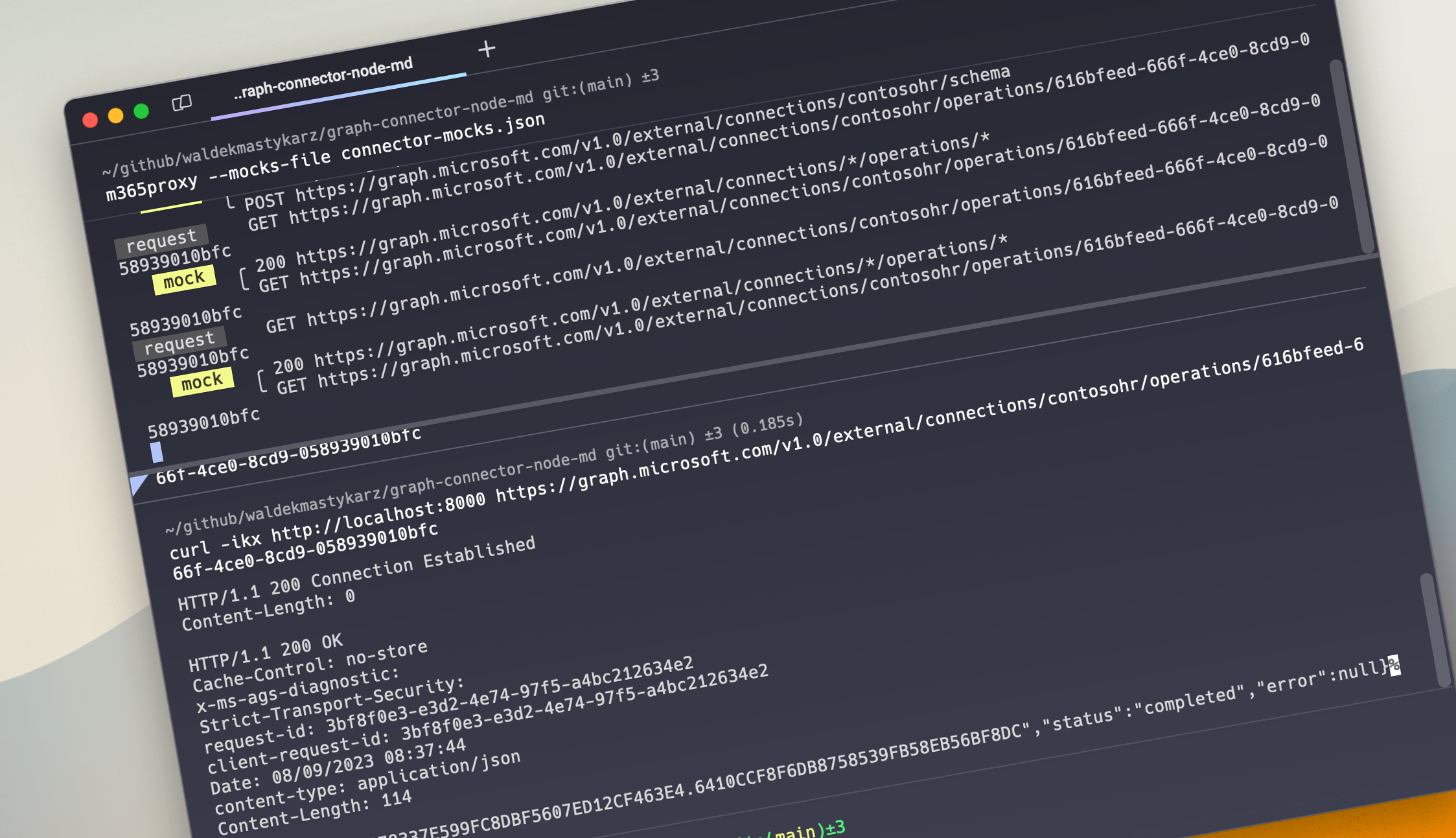
Mock nth request with Microsoft 365 Developer Proxy
Recently, we’ve updated Microsoft 365 Developer Proxy to allow you to mock n-th request, which means that you can now more easily simulate scenarios that consist of several API requests.
Test the untestable
Microsoft 365 Developer Proxy allows you to test how your app handles API errors. Without having to change your code, you can see in a matter of minutes how your app responds when its API requests get throttled or when it’s about to exceed rate limit. And you can use it with any app on any platform.
One of the features of Developer Proxy is the ability to mock API responses. Mocks are great for several reasons. Using mocks you will:
- easily test different scenarios without having to create the actual data
- more easily prepare demos that require time-sensitive data. Ever wanted to show an app that shows upcoming meetings, only to have to create dummy meetings last-minute?
- more easily prepare demos that require activity data. Have you built an app that shows your recent file, and had to scramble to open some files to generate some activity?
- test your code against a predefined API response, which is invaluable for running test locally but also on CI/CD
Mocking n-th request
Mocking a single API request isn’t hard. You specify the request that you’d like to mock, and define the response that your app should receive. That’s it. It gets more difficult, when you want the n-th request to the same API endpoint send a different response.
A typical scenario for having to mock n-th request are long-running operations. One example on Microsoft Graph is provisioning the schema of a Microsoft Graph connector. After you submit the schema, Microsoft Graph responds with the 202 Accepted
status, and a Location
header with the URL that you can call to get the status of the long-running operation.
POST https://graph.microsoft.com/v1.0/external/connections/contosohr/schema
Content-type: application/json
{
"baseType": "microsoft.graph.externalItem",
"properties": [
[...] trimmed for brevity
]
}
HTTP/1.1 202 Accepted
Location: https://graph.microsoft.com/v1.0/external/contosohr/operations/616bfeed-666f-4ce0-8cd9-058939010bfc
When you call the operations endpoint, you get the status of the provisioning process:
GET https://graph.microsoft.com/v1.0/external/contosohr/operations/616bfeed-666f-4ce0-8cd9-058939010bfc
HTTP/1.1 200 OK
Content-Type: application/json
{
"id": "616bfeed-666f-4ce0-8cd9-058939010bfc",
"status": "inprogress",
"error": null
}
The status
property will remain inprogress
as long as the long-running operation is running. When it completes, it’ll change to either completed
or failed
.
Depending on the operation, current server load, and your polling interval, it’ll take several requests before the job will complete. The provisioning process can take up to several minutes, so you can imagine, that if you’re writing code for handling long-running operations, you’ll waste a lot of time waiting for the operation to complete, when testing it on the actual API. Mocking the request is a great alternative that significantly improves the developer experience and saves you a lot of time.
Mocking n-th request using Microsoft 365 Developer Proxy
Without the ability to mock n-th request, simulating long-running operations is hard and requires modifying the operation URL to force different outcomes. But check out how easy it becomes now that we support simulating n-th request in Microsoft 365 Developer Proxy.
Note: we’ve introduced support for mocking n-th request in Microsoft 365 Developer Proxy v0.12.0-beta.1.
Here’s a sample Microsoft 365 Developer Proxy mock file that simulates the process of creating a Microsoft Graph connector and its schema (which is a long-running operation):
{
"responses": [
{
"url": "https://graph.microsoft.com/v1.0/external/connections",
"method": "POST",
"responseCode": 201,
"responseBody": {
"id": "contosohr",
"name": "Contoso HR",
"description": "Connection to index Contoso HR system",
"state": "ready"
}
},
{
"url": "https://graph.microsoft.com/v1.0/external/connections/*/schema",
"method": "POST",
"responseCode": 202,
"responseHeaders": {
"Location": "https://graph.microsoft.com/v1.0/external/connections/contosohr/operations/616bfeed-666f-4ce0-8cd9-058939010bfc"
}
},
{
"url": "https://graph.microsoft.com/v1.0/external/connections/*/operations/*",
"method": "GET",
"nth": 2,
"responseCode": 200,
"responseBody": {
"id": "1.neu.0278337E599FC8DBF5607ED12CF463E4.6410CCF8F6DB8758539FB58EB56BF8DC",
"status": "completed",
"error": null
}
},
{
"url": "https://graph.microsoft.com/v1.0/external/connections/*/operations/*",
"method": "GET",
"responseCode": 200,
"responseBody": {
"id": "1.neu.0278337E599FC8DBF5607ED12CF463E4.6410CCF8F6DB8758539FB58EB56BF8DC",
"status": "inprogress",
"error": null
}
}
]
}
The first mock, simulates the initial response for creating a new Graph connector (also known as external connection). Next, we simulate the 202 Accepted
response for submitting the schema and simulating the long-running operation of its provisioning. Then, we use the new nth
property, to define, that the second time Microsoft 365 Developer Proxy will intercept a request to the /operations
endpoint, it should respond with a success. The last mock defines the response for all other requests to the /operations
endpoint. When configuring mocks, we need to put mocks with the nth
property first, because they’re more specific and Developer Proxy uses mocks based on the first match.
Let’s see the result in practice. For simplicity, we’ll call Microsoft Graph APIs using curl.
We start with creating the Graph connector. Since we’re mocking the request anyway, we’ll skip the connector definition in the request body:
Next, we call Microsoft Graph to provision the connector’s schema. Again, for brevity, we’ll skip the schema definition:
When you look at the response, you’ll see the Location
header with the long-running operation status URL, all simulated by Microsoft 365 Developer Proxy. Let’s call it, to verify the status of the provisioning operation:
Because we’re calling the /operations
endpoint for the first time, Microsoft 365 Developer Proxy skips the nth=2 mock and uses the generic mock that we defined as last, telling us that provisioning the schema is still in progress. Let’s call the /operations
endpoint again:
As we’re calling the /operations
endpoint for the second time, Developer Proxy matches the nth=2 mock with the second request and takes precedence over the generic mock, and return a completed response.
You’ve just seen, how with a simple mock file and Microsoft 365 Developer Proxy, we’ve been able to simulate a complex API scenario. If you were building an app with similar API requests, you’d be able to easily test if it’s working as expected without having to call Microsoft Graph API and each time wait for the connector to be created and provisioned, and cleaned up, which is an additional long-running operation that adds even more time to your development time! By simulating API responses, you wait less and spend more time developing.
Summary
Using Microsoft 365 Developer Proxy you can easily simulate different API behaviors like throttling or rate limiting and verify how your app handles them. This allows you to build more robust apps and deliver awesome user experience to your customers. By mocking API responses, you can easily test specific scenarios, and with the recent addition of mocking nth request in the Microsoft 365 Developer Proxy, you can easily test scenarios that consist of multiple API calls such as long-running operations. Mocking APIs allows you to spend less time on setting up the test data and more time developing.