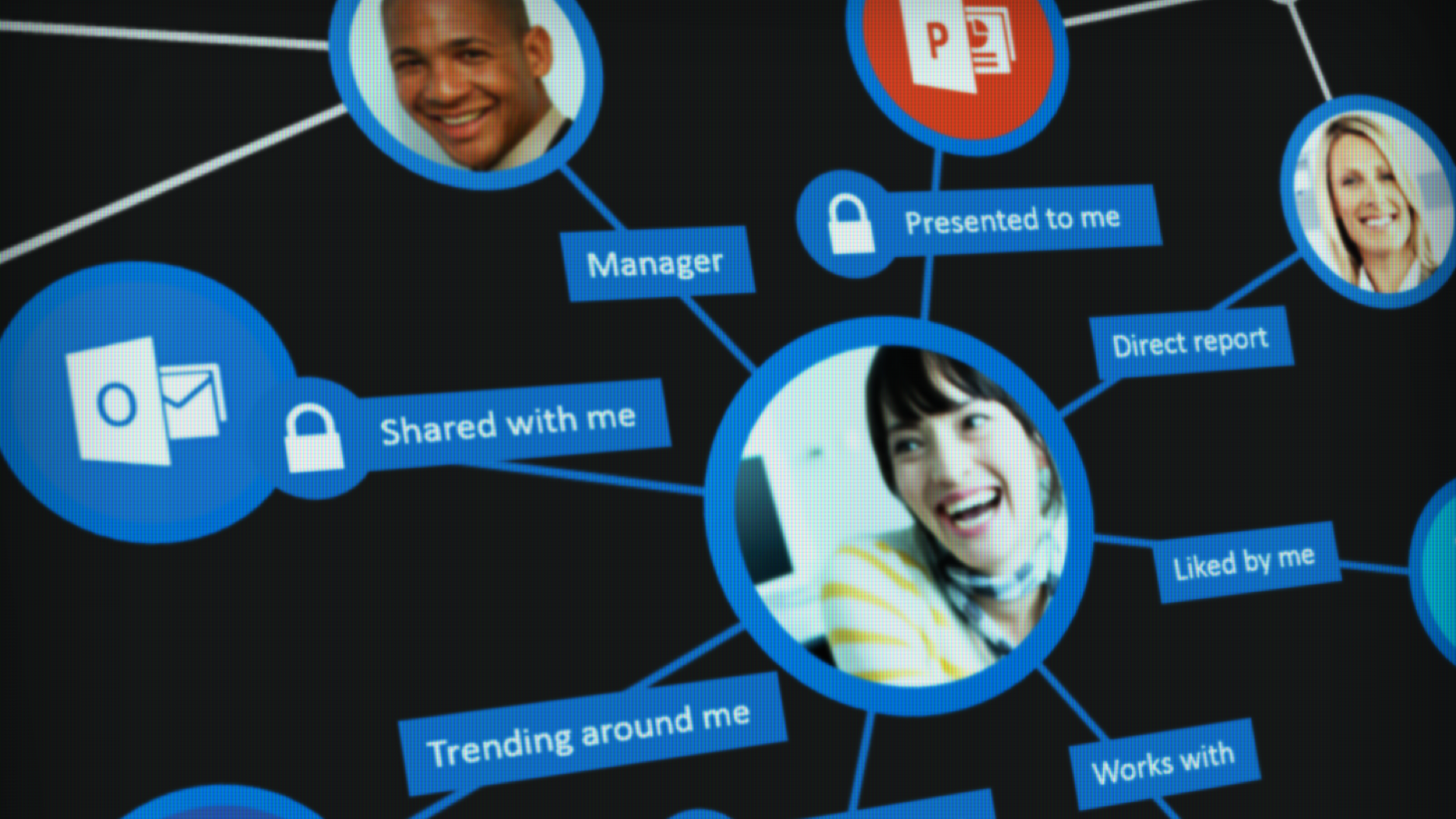
Query the Office Graph in SharePoint Framework client-side web parts
Using the Office Graph you can retrieve information and insights that help your users be more productive. To show you how to do this in SharePoint Framework client-side web parts, SharePoint Patterns and Practices published a number of sample web parts.
Increase business productivity with the Office Graph
Many organizations use Delve to discover relevant information trending in their organization. Through Delve users can easily see what is happening in their organization and what pieces of information are likely to help them in their current activities.
Delve is powered by the Office Graph: an intelligent fabric that keeps track of what you work on and with whom you work. Using these pieces of information it builds a network of information that it uses to provide you with personalized insights relevant to your work. All information stored inside the Office Graph is security-trimmed and no one else but you can access the insights it gathered about you.
Delve exposes only some insights available in the Office Graph. By querying the Office Graph you can support additional scenarios not available through Delve. Since the release of Delve, the way to communicate with the Office Graph is through the SharePoint Search API using the Graph Query Language (GQL). By extending SharePoint search queries with additional parameters you can retrieve personalized insights from the Office Graph.
By leveraging the power of the Office Graph you can build solutions that provide your users with contextual insights helping them complete their work.
Sample SharePoint Framework client-side web parts communicating with the Office Graph
To illustrate how to communicate with the Office Graph from SharePoint Framework client-side web parts, SharePoint Patterns and Practices recently published a number of sample web parts demonstrating the different integration scenarios with the Office Graph.
The source code of the sample web parts is available on GitHub at https://github.com/SharePoint/sp-dev-fx-webparts/tree/master/samples/react-officegraph.
Trending in this site
When visiting new sites, it’s hard for users to tell whether the particular site is relevant to them or not. Until recently SharePoint offered no insights into what is currently happening in the current site. When searching for information users have to manually browse through the different documents to see if what they are looking for is in that particular site or not, which is time-consuming and inefficient.
The ‘Trending in this site’ client-side web part uses the Office Graph to display documents trending in the particular site. Using the public insights stored in the Office Graph, the graph API returns a list of documents that members of that site have been recently working on. This web part help visitors to this site quickly see what is happening in that site which helps them decide whether that site is relevant for their research or not.
Working with
In our work we communicate with many different people. The bigger the organization the more complex the communication lines become. And if you’re involved with activities spanning multiple departments it might be hard for you to keep track of who the different people are and where in your organization they work.
The ‘Working with’ client-side web part uses the Office Graph to show you the list of individuals with whom you have been recently working. Office Graph keeps track of your recent Skype meetings, calendar meetings and emails. Using the Office Graph API you can retrieve private insights generated based on your activity and quickly see who these individuals are and how to get in touch with them.
At the moment of writing this article, clicking on the particular person takes you to her personal profile page. You could easily extend the web part with other capabilities such as starting a Skype meeting or contacting the particular person via e-mail.
My recent documents
The different Office client applications offer you a quick access to the documents recently opened in that particular application. But what if you’ve been working with different types of documents and would like to easily open them and switch between them?
The ‘My recent documents’ web part uses the Office Graph to retrieve the list of documents that you have recently been working with. If you have edited a document or merely viewed it, it will appear in the list allowing you to quickly open it no matter where it’s stored or what format it is. This web part improves your productivity if you frequently work with different documents stored in different locations across your intranet.
Trending in the sites I follow
SharePoint allows you to follow sites. Unfortunately following a site doesn’t offer you any particular benefits. What if however, you could easily stay up-to-date of everything that is happening in the sites you follow?
The ‘Trending in the sites I follow’ web part shows you an overview of documents that have been recently been used, stored in the sites you follow. From a single dashboard you get an overview of what is happening in all the sites you keep track of. If a particular document is interesting to you, you can access it with a single click directly from the web part.
Things you should have a closer look at
Using the Office Graph allows you to build powerful solutions that provide your users with contextual insights relevant to their work. The source code of all the web parts mentioned above is available on GitHub and I would encourage you to have a look at it. Here are some things that you should pay attention to in particular.
Communicate with the Office Graph using GQL
The only documented way of communicating with the Office Graph today is using the Graph Query Language. GQL can be used in combination with search queries issued through the SharePoint Search API. The GQL documentation on MSDN describes the basics how you can use it and the sample code shows you how you can apply it in practice.
From sites to insights
Office Graph network is built around people. Following the activity of the different users, the Office Graph builds a network of relationships between users (actors) and documents, linked with relationships called edges. Sites are not modeled inside the Office Graph and in order to retrieve insights for a particular site, you have to get a list of users related to that site. One way to do that is to retrieve the list of members of the particular site, by calling:
/_api/Web/AssociatedMemberGroup/Users?$select=Email
The site members API returns the list of e-mail addresses, but the Office Graph requires actor IDs in its queries. Actor IDs represent users in the search index. For each user you can retrieve her actor ID by issuing a people search query:
let query: string = '';
siteMembers.forEach((member: string): void => {
if (query.length > 0) {
query += ' OR ';
}
query += `UserName:${member}`;
});
If the particular site has many members, the search query might get too long to be executed using a GET request. To avoid any problems you should use a POST request instead:
const postData: string = JSON.stringify({
'request': {
'__metadata': {
'type': 'Microsoft.Office.Server.Search.REST.SearchRequest'
},
'Querytext': query,
'SelectProperties': {
'results': ['DocId', 'WorkEmail']
},
'RowLimit': '100',
'StartRow': '0',
'SourceId': 'b09a7990-05ea-4af9-81ef-edfab16c4e31'
}
});
return new Promise<string[]>((resolve: (actors: string[]) => void, reject: (err?: any) => void): void => {
component.request(`${siteUrl}/_api/search/postquery`, 'POST', {
'Accept': 'application/json;odata=nometadata',
'Content-Type': 'application/json;odata=verbose',
'X-RequestDigest': requestDigest
}, postData).then(
//...
);
});
Simplify code by chaining promises
Retrieving the list of documents trending in a particular site requires a number of subsequent REST calls. Information retrieved in one REST request is used in another. To simplify the code you can chain the promises returned by the different request together.
Working with search results in client-side web parts
By default you use TypeScript when building SharePoint Framework client-side web parts. One benefit of using TypeScript over plain JavaScript are the typesafety features that help you discover inconsistencies in your code already during development.
When communicating with SharePoint Search using the REST API, it returns its results in a complex structure. Retrieving the contents from that structure is more complex than when working with other SharePoint APIs. Graph Query is available through the SharePoint Search API and the different web parts illustrate how you can process the response of a the SharePoint Search API in your client-side web parts, at the same time benefiting of TypeScript’s typesafety features.
Summary
Using the Office Graph you can retrieve information and insights that help your users be more productive. To show you how to do this in SharePoint Framework client-side web parts, SharePoint Patterns and Practices published a number of sample web parts. These web parts illustrate using the Graph Query Language in practice as well as a number of other concepts applicable to building web parts that use the SharePoint Search REST API.
Check out the sample SharePoint Framework client-side web parts connected to the Office Graph on GitHub at https://github.com/SharePoint/sp-dev-fx-webparts/tree/master/samples/react-officegraph.