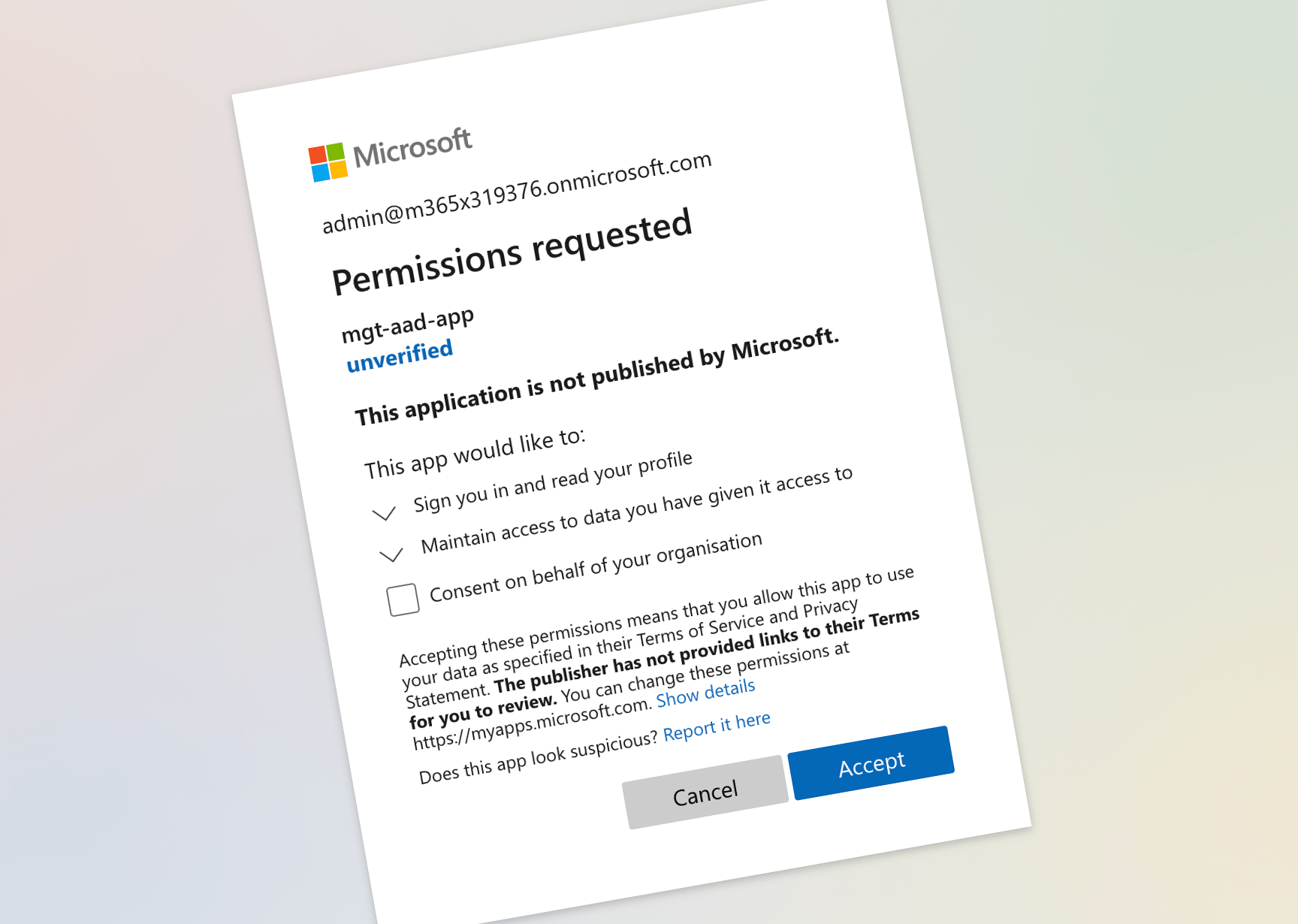
Preconsent multiple scopes with Microsoft Graph Toolkit
When working with Microsoft Graph Toolkit you might want to preconsent multiple scopes upfront to improve the user experience. Here’s how to do it.
The easiest way to connect your app to Microsoft 365
Using Microsoft Graph Toolkit is the easiest way to connect your app to Microsoft 365.
If you want to connect your app to Microsoft 365, you need to take care of several things. First, you need to let users sign in to your app with their Microsoft 365 account. Then, you need to get an access token. Next, you need to build a request to Microsoft Graph - the API that lets you work with data and insights stored in Microsoft 365. When the request is ready, you need to execute it and handle its response. Typically you will get the data you requested, but it could also be an exception that you need to handle as well. Finally, you need to show the data in your app. Sounds like a lot doesn’t it
Microsoft Graph Toolkit handles all of this for you with its web components and authentication providers. Instead of working on the plumbing, you can focus on building your app instead! How’s that for a productivity boost?!
Request access to what you need
When building apps connected to Microsoft 365, you might need access to different pieces of information.
Say, that after signing users into your app, you want to show their account information. In another place in your app, you want users to see their upcoming meetings. Each of these operations requires your app to have different permissions (also referred to as scopes) to data in Microsoft 365 (user profile vs. calendar). While the account information is visible to users directly after signing in, they might not use the part of your app that shows upcoming meetings. As such, your app shouldn’t request access to their calendar, until users use that part of your app.
This concept is named incremental consent and is considered a best practice when building apps connected to Microsoft 365. In your apps, you should request minimal permissions to access user data at first. You should request additional permissions when users get to work with other areas in your app that load specific information from Microsoft 365.
Microsoft Graph Toolkit follows this approach in its components. Each component knows which data in Microsoft 365 it works with and which permissions it requires to access it. And while components require the necessary permissions dynamically, sometimes you might want to request a set of permissions upfront.
Incremental consent with Microsoft Graph Toolkit in action
Say you build a single page app, that lets users sign in with their Microsoft 365 account and view upcoming meetings. You’d use the following code:
<html>
<head>
<script src="https://unpkg.com/@microsoft/mgt@2/dist/bundle/mgt-loader.js"></script>
</head>
<body>
<mgt-msal2-provider client-id="c35a6a0b-7275-4265-a267-d33757115fdc"></mgt-msal2-provider>
<mgt-login></mgt-login>
<mgt-agenda></mgt-agenda>
</body>
</html>
When you launch the app, select the Sign in button, and sign in with your Microsoft 365 account, you will be prompted to consent to the app the access to your user profile.
After granting the app access, the app continues loading… only to show you another consent, and another one, and another one. And if you hover over one of the meetings’ attendees, you will see another consent!
This is incremental consent in action. As components load their data and need additional permissions, they prompt the user with additional consent requests. But it’s hardly what you’d call a great user experience. So what can you do about it?
Preconsent multiple permissions in Microsoft Graph Toolkit
When using Microsoft Graph Toolkit in your app, rather than following the default experience that you have just seen, you can preconsent multiple scopes upfront. Because you know your application, you know which toolkit components are used on the landing page and which permissions they will need. By requesting consent for these permissions upfront you can show the user a single consent and significantly improve their experience. So how to do it?
To preconsent multiple permissions in Microsoft Graph Toolkit, extend the provider definition with the scopes
attribute and specify a comma-separated list of the permissions that you’d want to request:
<html>
<head>
<script src="https://unpkg.com/@microsoft/mgt@2/dist/bundle/mgt-loader.js"></script>
</head>
<body>
<mgt-msal2-provider client-id="c35a6a0b-7275-4265-a267-d33757115fdc" scopes="user.read, user.readbasic.all, people.read, presence.read.all, presence.read, calendars.read, user.read.all, contacts.read, sites.read.all, mail.readbasic, people.read.all"></mgt-msal2-provider>
<mgt-login></mgt-login>
<mgt-agenda></mgt-agenda>
</body>
</html>
Tip: each Microsoft Graph Toolkit component requires different scopes. Some components also use other components internally. To find out which permissions each component requires, see its documentation.
The app you’ve just seen uses the login and the agenda component. Internally, they use the person, people and person card components. By combining the scopes of all these components, you get a list of all scopes that you should request upfront. By listing them in the provider’s scopes
attribute, you show the user a single consent prompt which significantly improves their experience.
The scopes
attribute is meant to help you request an initial set of permissions for your app. If you have another page in your app using different components, which require additional permissions, they will use the incremental consent and prompt users to grant the necessary permissions when they navigate to that page.
Summary
Microsoft Graph Toolkit significantly simplifies connecting apps to Microsoft 365. With just a few lines of code, you will be able to let users sign in to your app using their Microsoft 365 account and interact with their data stored in Microsoft 365. If you want to know more about Microsoft Graph Toolkit, check out the Microsoft Graph Toolkit learning path on MS Learn.