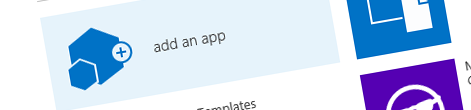
Programmatically working with Lists and Libraries using the App Model
In the previous article we discussed how to leverage the new App Model for provisioning Site Columns and Content Types. In this article we will look into working with Lists and Libraries.
Creating Lists and Libraries
In order to create a List or a Library using the App Model your app requires the Manage permission on the Web where the List will be created.
The process of creating a List is straight-forward and requires you to construct a ListCreationInformation object that contains all information about the List that you want to create:
Web web = clientContext.Web;
web.Lists.Add(new ListCreationInformation {
Title = "Sessions",
Description = "SPSNL 14 Sessions",
Url = "Lists/Sessions",
TemplateType = (int)ListTemplateType.GenericList,
TemplateFeatureId = new Guid("00BFEA71-DE22-43B2-A848-C05709900100"),
});
clientContext.ExecuteQuery();
The important parameters are the TemplateType and TemplateFeatureId which specify which template you want to use to create your List and which Feature has that template been deployed with.
Tip: If you’re referring to any of the standard SharePoint List types, you can use the ListTemplateType enumeration to specify the type of the List you wish to create. This will make you code easier to read.
Tip #2: If the TemplateType is set using an integer, you can easily track if the specified List Template is a standard SharePoint List Template or not. If you look at the GUID specified in the TemplateFeatureId property many of the standard SharePoint List Templates are provisioned by Features that begin with 00BFEA (Out-Of-the-Box FEAture) and end with the integer number of the List Template (100 in the example above which is the number of the Custom List Template).
Associating Content Types with Lists
Once the List is created the next step is to associate your custom Content Types with it. Before you will be able to do that however, often you will first need to enable support for Content Types on the List. Only after that you will be able to add your Content Types to the List.
clientContext.Load(clientContext.Web, w => w.ServerRelativeUrl);
clientContext.ExecuteQuery();
Microsoft.SharePoint.Client.List sessionsList = clientContext.Web.GetList(String.Format("{0}/Lists/Sessions", clientContext.Web.ServerRelativeUrl.TrimEnd('/')));
ContentType sessionContentType = clientContext.Web.AvailableContentTypes.GetById("0x0100BDD5E43587AF469CA722FD068065DF5D");
sessionsList.ContentTypesEnabled = true;
sessionsList.ContentTypes.AddExistingContentType(sessionContentType);
sessionsList.Update();
clientContext.ExecuteQuery();
The process starts with retrieving the List. Using Client-Side Object Model (CSOM) you can easily retrieve the List using its Title or ID. The problem with using the Title is that, as it can be changed by users, it’s not a reliable value to use in structured and repeatable deployments. And as IDs are auto-generated by SharePoint and not known to us, they are not a suitable method either. A more reliable approach is to retrieve the List by its URL which is specified when creating the List and which cannot be changed afterwards. For this however you will need the server-relative URL of the Web where the List is to be created (line 1). With this, you can call the Web.GetList method passing the server-relative URL of the List (line 4).
The next step is to retrieve the Content Type. In order to ensure that you will look for the Content Type that you want to add within all Content Types available in your Site Collection, and not only those created in your Site, you should use the Web.AvailableContentTypes property to retrieve your Content Type (line 5). As described in more detail in the previous article, depending on how you chose to create your Content Type you will be able to retrieve it either by its ID or Name.
As mentioned earlier, before you will be able to add a Content Type to a List, you need to ensure that the List supports Content Types. You can easily enable Content Types on a List by setting the List.ContentTypesEnabled property to true (line 7).
The only thing left to do, is to add the Content Type to the List by calling the List.ContentTypes.AddExistingContentType method passing the reference to the Content Type as a parameter.
Choosing which Content Types should be available in the List
When working with custom Content Types often the requirement is to allow users to create content using those Content Types only. To fulfill this requirement, all standard Content Types should be removed from the List.
clientContext.Load(clientContext.Web, w => w.ServerRelativeUrl);
clientContext.ExecuteQuery();
Microsoft.SharePoint.Client.List sessionsList = clientContext.Web.GetList(String.Format("{0}/Lists/Sessions", clientContext.Web.ServerRelativeUrl.TrimEnd('/')));
var standardContentTypes = clientContext.LoadQuery(sessionsList.ContentTypes.Where(ct => ct.Name != "Session"));
clientContext.ExecuteQuery();
foreach (ContentType ct in standardContentTypes) {
ct.DeleteObject();
}
sessionsList.Update();
clientContext.ExecuteQuery();
Similarly to the previous step, the process begins with retrieving the List. Next, you should get the list of all Content Types that you want to remove from the List. Depending on your scenario you might pass the name of your Content Type and retrieve all other Content Types, as we do in the example above, or you could explicitly state which Content Types you want to remove from the List. Either way, after executing the query you should get a collection of Content Types to be removed. By iterating through that collection and calling the ContentType.DeleteObject method on each Content Type, you will remove them from the List.
Creating List View
One more thing that you are very likely to do when working with custom Content Types and Lists is to create a List View to include your custom Columns.
clientContext.Load(clientContext.Web, w => w.ServerRelativeUrl);
clientContext.ExecuteQuery();
Microsoft.SharePoint.Client.List sessionsList = clientContext.Web.GetList(String.Format("{0}/Lists/Sessions", clientContext.Web.ServerRelativeUrl.TrimEnd('/')));
sessionsList.Views.Add(new ViewCreationInformation {
Title = "Sessions",
ViewTypeKind = ViewType.Html,
ViewFields = new string[] { "Title", "SessionName" },
SetAsDefaultView = true,
RowLimit = 25,
PersonalView = false,
Paged = true
});
clientContext.ExecuteQuery();
Creating a List View is relatively easy and comes down to providing a ViewCreationInformation object that contains all the necessary information to create a List View. The properties on the ViewCreationInformation object are self-explanatory and very similar to the parameters you would use when programmatically creating List Views using the server-side API.
Download: Sample App for SharePoint (227KB, ZIP)