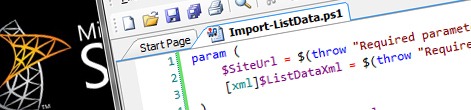
Provisioning List Instance data using PowerShell
Recently I showed you how you can import List Instances and their data to your SharePoint Project so that you can reuse test data during the development process. If however creating test Features is too much work for you and you are more of a PowerShell guy this article might be just the right thing for you. Find out how to provision List Instance data using PowerShell.
Reusing test data
Importing List Instance data into your project allows you to reuse your test data during the whole development process. This not only saves you time but also helps you improve the quality of your solution by testing the solution with real-life data.
Using the Mavention Import List Instance extension importing a List Instance and its data is a matter of a few mouse clicks. And by removing all the unwanted fields you can keep your test data files clean and manageable.
In my previous articles I mentioned that you could provision imported List Instances using Features. By creating separate SharePoint Packages (WSP) you could separate the test data from the real Solution and make provisioning test data a part of your build process during the development and maybe even the test stage.
Creating SharePoint Packages and Features introduces overhead. And even though the Visual Studio 2010 SharePoint Developer Tools make it very easy to us it still might be too much work for you to create and maintain those artificial deliverables. So if you posses a different kind of skills such as PowerShell there might an even easier way to provision List Instance data during the provisioning process.
Provisioning List Instance data using PowerShell
If you want to provision List Instance data using PowerShell all you need is the following PowerShell script:
param (
$SiteUrl = $(throw "Required parameter -SiteUrl missing"),
[xml]$ListDataXml = $(throw "Required parameter -ListDataXml missing")
)
$web = Get-SPWeb $SiteUrl
# get prerequisites
$listUrl = $ListDataXml.Elements.ListInstance.Url
if (-not($listUrl)) {
throw "List URL missing in <ListInstance Url='...'/>"
}
$list = $web.GetList($listUrl)
Write-Host "Importing data for list '$($list.Title)'..."
$i = 0
foreach ($row in $ListDataXml.Elements.ListInstance.Data.Rows.Row) {
$i++
Write-Host "`tProcessing item $($i)..."
$item = $list.AddItem()
foreach ($field in $row.Field) {
Write-Host "`t`tField $($field.Name)..." -NoNewLine
$listField = $list.Fields.GetFieldByInternalName($field.Name)
if ($listField.Type -eq "DateTime") {
$item[$field.Name] = [DateTime]::Parse($field.InnerText, $web.Locale, "AssumeUniversal")
}
else {
$item[$field.Name] = $field.InnerText
}
Write-Host "DONE" -ForegroundColor Green
}
$item.Update()
}
Write-Host "`Data import completed" -ForegroundColor Green
Assuming you called the PowerShell script file Import-ListData.ps1 you could call the script as follows:
[xml]$listDataXml = Get-Content .\Elements.xml
.\Import-ListData.ps1 http://www.sharepoint.local $listDataXml
The first parameter is the URL of your site where the List is located. The second parameter contains the XML contents of the Elements.xml file created while importing the List Instance.
How it works
The script starts with retrieving the Site where the List is located (line 6). Next, using the List URL from the Elements.xml file (line 9), the List is being opened (line 14). Finally for every Row element in the Elements.xml file, a new List Item is being added (line 22) and filled with data from the XML file (lines 24-37).
When filling items with data there is one important thing to keep in mind. SharePoint expects DateTime values to be provided as Universal Time. While this doesn’t matter that much when setting values programmatically, this is the format that SharePoint expects when provisioning List Instance data using Features. So in order to keep the data interchangeable also the script uses the Universal Time format (line 30).
And that’s it: importing List Instance data using PowerShell.