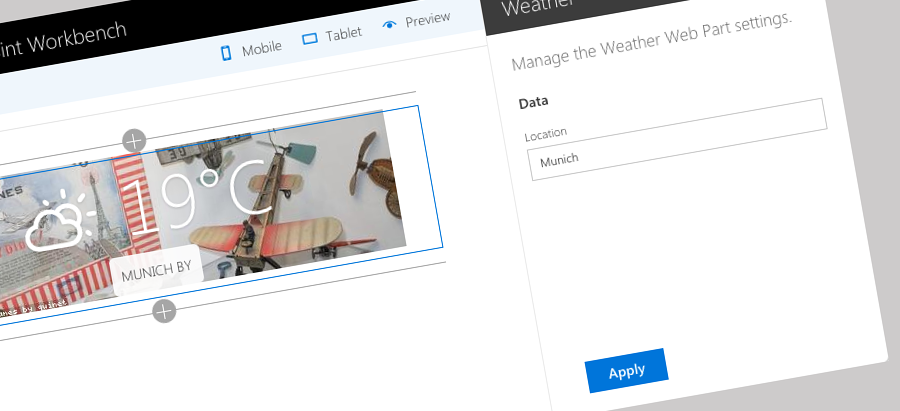
Building SharePoint Framework Client Side Web Parts with non-reactive property pane
Seeing the changes to your Web Part properties immediately is great, but is it what you really want for every single scenario?
Web Part properties yesterday
In the past, when working with Web Parts, whenever we wanted to change the configuration of a Web Part, we would go to the Web Part’s settings, change the configuration and click the Apply button to have the Web Part reflect our changes. If you weren’t sure about the desired configuration and wanted to try out a couple of options, clicking the Apply button to preview each change was tedious.
SharePoint Framework Client Side Web Part properties
When working with SharePoint Framework Client Side Web Parts, each change of a Web Property is directly visible in the Web Part. You change the title and it changes in the Web Part - as you type.
This is called a reactive property pane.
While it seems awesome, imagine that you wanted to configure the data source and the Web Part would try to load the data on every keystroke of you changing the value:
As you see the reactive property pane isn’t the best option for all scenarios either. Just as the Apply button in the past, it’s nice for some scenarios, but less useful for others. What if we could control how we want the property pane to behave…
Configuring property pane behavior in SharePoint Framework Client Side Web Parts
Where in the past the behavior of the property pane was fixed, the SharePoint Framework allows us to specify whether we want the property pane for our Client Side Web Part to automatically refresh the Web Part on each property change (reactive) or not (non-reactive).
By default the property pane is reactive. If you want to change it non-reactive, all you have to do is to add the disableReactivePropertyChanges
function to your Web Part class and have it return true
:
export default class HelloWorldWebPart extends BaseClientSideWebPart<IHelloWorldWebPartProps> {
public constructor(context: IWebPartContext) {
super(context);
}
public render(mode: DisplayMode, data?: IWebPartData): void {
// omitted for brevity
}
protected get propertyPaneSettings(): IPropertyPaneSettings {
// omitted for brevity
}
protected get disableReactivePropertyChanges(): boolean {
return true;
}
}
When you do that, the next time you edit your Web Part properties you will see the Apply button. The Web Part will reflect changes to its properties only after you click the Apply button.
Summary
When building Client Side Web Parts using the SharePoint Framework you can choose whether the Web Part should automatically update on each change of its properties or not. This behavior can be controlled using the disableReactivePropertyChanges property in the Web Part’s code.