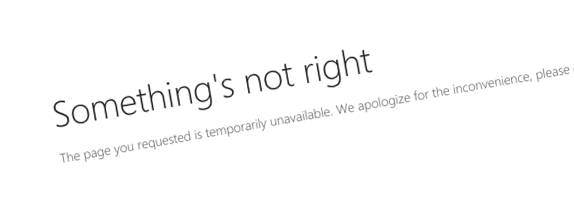
Simulating throttling in SharePoint
When you communicate with SharePoint using its APIs, you have to assume that you might get throttled. Here is how to verify if your application can handle it correctly.
Two sides of remote SharePoint APIs
Back in the days, when we used to build SharePoint solutions, we were writing code that would run on the SharePoint servers. Because our code ran next to SharePoint, we didn’t need to worry about connectivity or network issues.
When Microsoft started working on hosting SharePoint as a service, they noticed, that deploying custom code to SharePoint servers is risky. Not only it impacts everything running on that server, but worst case, if the code doesn’t run as intended, it can bring the whole farm down.
So to offer us an alternative, Microsoft started investing in remote APIs: Client-Side Object Model (CSOM) and REST APIs that we can use to communicate with SharePoint while having our code run not on SharePoint servers. Undoubtedly, moving custom code away from SharePoint servers allows for extending SharePoint Online. But even if you host SharePoint on-premises, you still benefit of increased platform hygiene and easier upgrade path. But all this comes at a price.
429 TOO MANY REQUESTS or throttling in SharePoint
Microsoft hosts SharePoint as a service for many organizations. Every SharePoint Farm hosts many SharePoint tenants that often belong to different organizations. Each organization is Microsoft’s customer and as such, has right for a reliable collaboration service - SharePoint. So to deliver on their promise, Microsoft monitors traffic to the different tenants. If any of the tenants shows anomalous activity, to prevent it from degrading the performance of other tenants on the same Farm, Microsoft will start throttling requests. Rather than getting regular responses, your API calls will start failing with 429 TOO MANY REQUESTS
responses.
There is no guaranteed approach for you to avoid throttling in your SharePoint application. Instead, you should always take into account that it might happen and you should ensure that your code handles it gracefully.
Handle throttling in SharePoint
When you get a 429 TOO MANY REQUESTS
response in SharePoint, you know that you’re being throttled. To stop throttling, you must temporarily decrease the number of requests you issue to SharePoint.
A part of a 429 response, is the Retry-After
header, which specifies the number of seconds which you should wait before issuing another request. For SharePoint, the default is 120 seconds, but you should retrieve this value directly from the response header, in case it changes in the future.
Now that you know how to handle throttling in SharePoint, how to verify that you’ve done it correctly?
Simulate throttling in SharePoint
When working with SharePoint and its APIs, you can simulate throttling by appending ?Test429=true
to the request URL. If you append it to a URL of a page, you will be redirected to an error page.
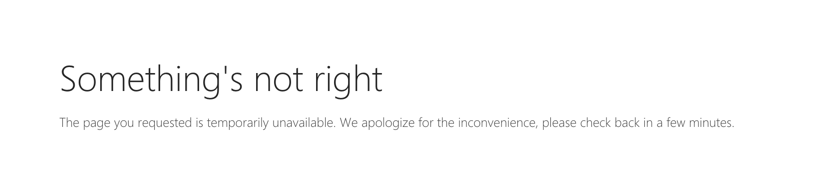
If you append it to an API call, either using REST or CSOM, you will get a 429 TOO MANY REQUESTS
response with the Retry-After header.
By simulating these responses, you can verify that your code handles throttling correctly and backs off for the period of time specified by SharePoint.
Automatically add the Test429 query string parameter to outgoing SharePoint requests
When working with SharePoint REST APIs, you could consider adding the Test429=true
query string parameter to the API calls yourself to simulate throttling. But this is not an option when working with CSOM. A better approach, one that doesn’t require you to change your code, is to use a proxy such as Fiddler, to append this query string parameter for you to specific outgoing requests.
To do that with Fiddler, from the Rules menu, select the Customize Rules… option.
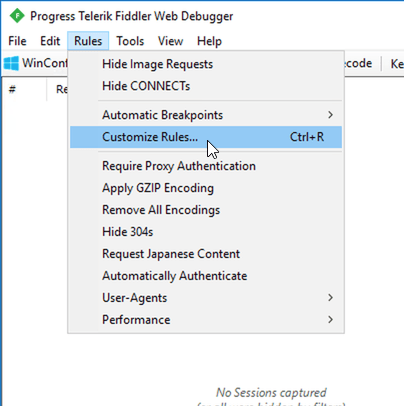
Next, in the Fiddler ScriptEditor, find the OnBeforeRequest
method, and add to it your rules. For example, to force throttling on all SharePoint REST API calls, add:
if (oSession.url.indexOf("contoso.sharepoint.com") > -1 &&
oSession.url.indexOf("/_api/") > -1) {
oSession.url = oSession.url + "?Test429=true";
}
To test throttling with CSOM calls, use instead:
if (oSession.url.indexOf("contoso.sharepoint.com") > -1 &&
oSession.url.indexOf("ProcessQuery") > -1 &&
oSession.RequestMethod == "POST") {
oSession.url = oSession.url + "?Test429=true";
}
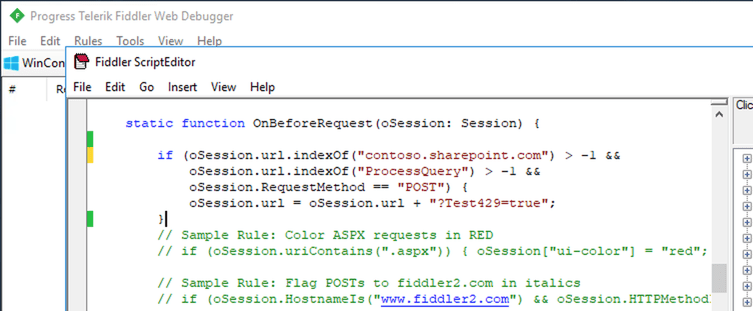
With this rule, any call to the SharePoint API will fail with a 429 TOO MANY REQUESTS response, similarly to how it would if you were throttled, allowing you to verify that your code can handle it appropriately.
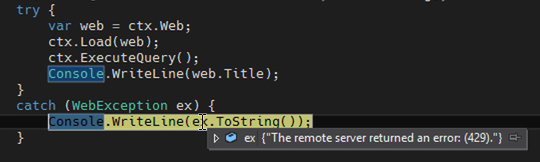