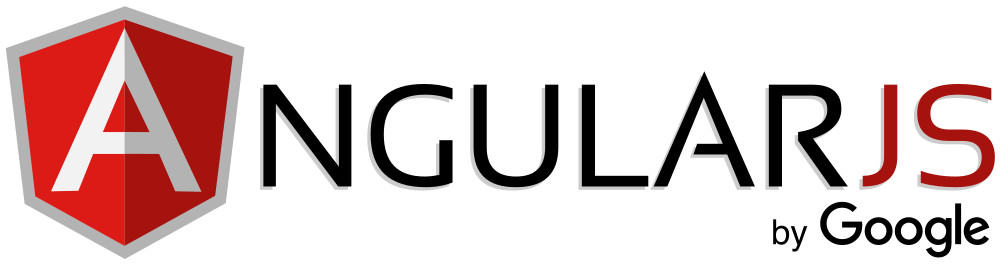
4 tips to improve performance of your AngularJS applications
4 tips to improve performance of your AngularJS applications
If you’re building AngularJS applications, here are 4 tips that you can use to improve their performance.
AngularJS - not quite done yet
While many developers have been building applications using Angular for a while now, AngularJS, also known as Angular v1.x, is still widely used. Not only are there many AngularJS applications running in production, but also new applications using AngularJS are built every day. Whether it’s due to the lack of knowledge of Angular or technical limitations, such as those that apply to building SharePoint Framework solutions, if you still work with AngularJS, check the application that you are currently working on, to see if there is room for improvement.
Improve performance of your AngularJS applications
Following are a few tips that can help you improve performance of your AngularJS applications. Applying them is relatively straight-forward. What’s surprising, is how few AngularJS samples on the Internet mention them.
Use the inline array annotation for dependency injection
AngularJS uses dependency injection for passing references to services and other objects. In the easiest setup, these references are automatically assigned, based on the name of function parameters, for example:
angular.module('app')
.controller('HomeController', HomeController);
function HomeController(myService) {
}
AngularJS uses the names of the parameters to determine which objects should be injected. If you minify your code, it could become similar to:
a.module('app').controller('HomeController',b);function b(c){}
At that point, the parameter previously called myService
has just become c
. As a result, AngularJS won’t know which object to inject into the controller and your application will fail on runtime.
To mitigate this issue, AngularJS allows you to use the inline array annotation for injecting dependencies, for example:
angular.module('app')
.controller('HomeController', ['myService', HomeController]);
function HomeController(myService) {
}
The controller constructor function is wrapped in an array and passed as the last array item. Preceding it, are strings with the names of objects that should be injected into the function. Using this construct, even if your code is minified, and the names of function parameters get changed, the strings remain unmodified and AngularJS uses them to assign the correct object references to function parameters based on their order. Using the inline array annotation is the recommended way of injecting dependencies in AngularJS.
Alternatively to using the inline array annotation, you can use the $inject
property to assign the array of strings to it. This is particularly useful when defining controllers as TypeScript classes where they cannot be wrapped in an array:
export default class HomeController {
public static $inject: string[] = ['myService'];
constructor(private myService: IMyService) {
}
}
The $inject
property can be used with plain JavaScript as well, and some find it easier to read than the inline array annotation:
angular.module('app')
.controller('HomeController', HomeController);
HomeController.$inject = ['myService'];
function HomeController(myService) {
}
Using explicit annotation (this is either using the inline array annotation or the $inject
property) to define the different resources to be injected has undoubtedly its benefits when minifying your code, but did you also know, that it improves the performance of your application? Getting the list of objects to inject from an array is more efficient than iterating through the different function parameters. As a result AngularJS can faster inject dependencies into your objects improving the performance of your application.
Enable strict DI mode
Defining which dependencies should be injected using either the inline array annotation or the $inject
property is optional. Especially if you haven’t been using it from the start of your project and you are working on a big application, it’s easy to miss, leading to hard-to-find errors.
AngularJS allows you to set the application in the strict DI mode. When set, the strict DI mode will cause AngularJS to throw an exception every time you’re using dependency injection without using explicit annotation to describe which dependencies should be injected.
If you’re bootstrapping your application using angular.bootstrap
, you can enable the strict DI mode by passing a third parameter into the bootstrap
function:
angular.bootstrap(document, ['app'], {
strictDi: true
});
If you prefer to use the ngApp
directive instead, you can enable the strict DI mode using the ng-strict-di
argument:
<div ng-app="app" ng-strict-di>
<!-- your app here -->
</div>
Using the strict DI mode is an easy way to verify, that you’re using explicit annotation consistently throughout your application. If you have automated tests passing through your whole application, you will quickly see if there are any exceptions related to incorrect dependency injection and you will be able to fix them improving the performance of your application.
Disable comment and CSS class directives
When building AngularJS directives, you can specify whether they can be used as an element, attribute, CSS class and comments. The first two types of directives are widely used. CSS class and comment directives were used mostly on occasions where including additional elements or attributes was not possible or desirable.
As AngularJS evolved over time, CSS class and comment directives became used less frequently. Yet, by default, AngularJS will try to resolve them in your application. If you are not using CSS class or comment directives and you are not using other libraries that rely on them, you can improve the performance of your AngularJS application by disabling support for CSS class and comment directives:
angular.module('app').config(['$compileProvider', configureModule]);
function configureModule($compileProvider) {
$compileProvider.commentDirectivesEnabled(false);
$compileProvider.cssClassDirectivesEnabled(false);
}
With CSS class and comment directives disabled, the AngularJS compiler will not spend time iterating through all CSS classes and comments in your templates searching for directives which will improve the performance of your application.
Disable debug data
When building AngularJS applications, it’s inevitable, that sooner or later you will stumble upon a bug in your application. By default, AngularJS attaches information about binding and scopes to DOM elements. Tools such as Batarang or Protractor use this information to help you debug your application. Once your application has been tested and runs as expected, adding all that extra information slows your application down. To optimize your application’s performance, you can disable the debug information using:
angular.module('app').config(['$compileProvider', configureModule]);
function configureModule($compileProvider) {
$compileProvider.debugInfoEnabled(false);
}
Disabling debug information should noticeably improve the performance of your AngularJS application.
Summary
AngularJS is a powerful library for building client-side applications. While many developers already started using Angular, there are still many AngularJS applications being actively worked on. If you’re building AngularJS applications, there are a few easy tips that you should keep in mind that will help you improve their performance.